top of page
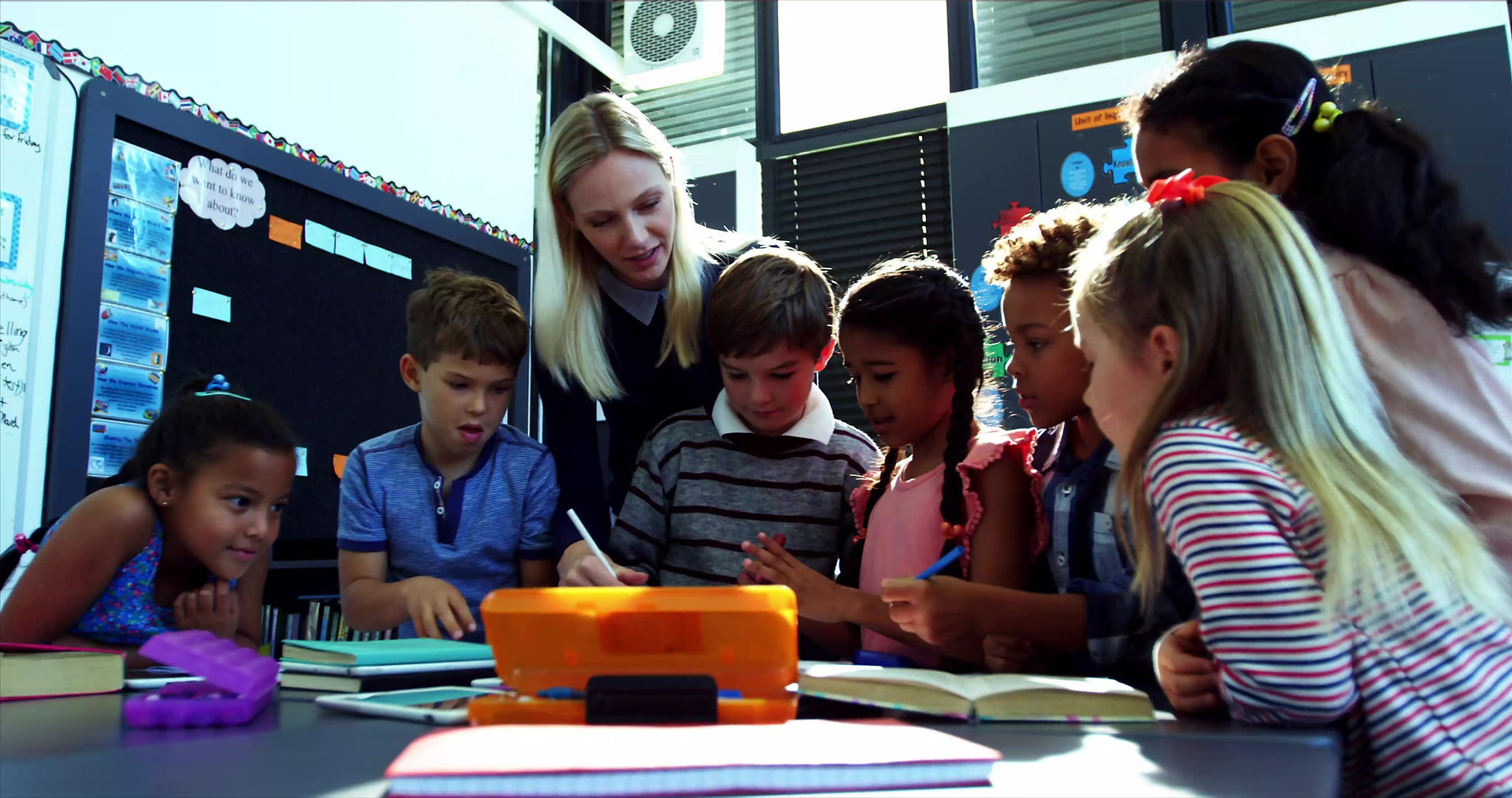
게시판 게시물
wadepark2020
2025년 1월 12일
In 소스 코드 제출
/*
#include <stdio.h>
int a[1000005]={};
int b[100001]={};
int i,j,n;
int bs(int start,int end,int k)
{
if(a[end]<k)
{
return -1;
}
int mid=(start+end)/2;
if(a[mid]==k)
{
return mid;
}
else if(k<a[mid])
{
return bs(start,mid-1,k);
}
else
{
return bs(mid+1,end,k);
}
}
int main()
{
int n,k;
scanf("%d",&n);
for(i=1;i<=n;i++)
{
scanf("%d",&a[i]);
}
scanf("%d",&k);
for(i=1;i<=k;i++)
{
scanf("%d",&b[i]);
}
for(i=1;i<=k;i++)
{
printf("%d ",bs(1,n,b[i]));
}
return 0;
}
*/
/*
#include <stdio.h>
int a[100005]={};
int i,j,n;
int bs(int start,int end,int k)
{
if(a[end]<k)
{
return end+1;
}
int mid=(start+end)/2;
if(a[mid]==k)
{
return mid;
}
else if(k<a[mid])
{
return bs(start,mid-1,k);
}
else
{
return bs(mid+1,end,k);
}
}
int main()
{
int k;
scanf("%d %d",&n,&k);
for(i=1;i<=n;i++)
{
scanf("%d",&a[i]);
}
if(a[n-1]<k)
{
printf("%d",n+1);
}
else
{
int s=bs(1,n,k);
while(s>1 && a[s]==a[s-1])
{
s--;
}
printf("%d",s);
}
return 0;
}
*/
/*
y=x+5
y에 0대입
-->0=x+5
x=-5
*/
/*
#include <stdio.h>
int a[1001]={};
int b[2500]={};
int i,j,n;
int bs(start,end,k)
{
if(a[end]<k)
{
return end+1;
}
int mid=(start+end)/2;
if(a[mid]==k)
{
return mid;
}
else if(a[mid]>k)
{
return bs(start,mid-1,k);
}
else
{
return bs(mid+1,end,k);
}
}
int main()
{
int k;
scanf("%d %d",&i,&j);
for(i=1;i<=n;i++)
{
}
}
*/
0
0
1
wadepark2020
2025년 1월 05일
In 소스 코드 제출
/*
#include <stdio.h>
typedef struct
{
int a;
int b;
int c;
}myint;
int main()
{
int i,j,n;
myint temp;
myint arr[1001];
scanf("%d",&n);
for(i=1;i<=n;i++)
{
scanf("%d %d",&arr[i].b,&arr[i].c);
arr[i].a=i;
}
for(i=1;i<n;i++)
{
for(j=1;j<=n-i;j++)
{
if(arr[j].b<arr[j+1].b)
{
temp=arr[j];
arr[j]=arr[j+1];
arr[j+1]=temp;
}
else if(arr[j].b==arr[j+1].b)
{
if(arr[j].c>arr[j+1].c)
{
continue;
}
else if(arr[j].c<arr[j+1].c)
{
temp=arr[j+1];
arr[j+1]=arr[j];
arr[j]=temp;
}
}
}
}
for(i=1;i<=n;i++)
{
printf("%d %d %d\n",arr[i].a,arr[i].b,arr[i].c);
}
return 0;
}
*/
/*
#include <stdio.h>
#include <string.h>
typedef struct
{
int b,c,d;
char a[100];
}myint;
int main()
{
int i,j,n;
myint temp;
myint arr[101];
scanf("%d",&n);
for(i=1;i<=n;i++)
{
scanf("%s %d %d %d",&arr[i].a,&arr[i].b,&arr[i].c,&arr[i].d);
}
for(i=1;i<=n;i++)
{
for(j=1;j<=n-i;j++)
{
if(arr[j].b>arr[j+1].b)
{
temp=arr[j];
arr[j]=arr[j+1];
arr[j+1]=temp;
}
else if(arr[j].b==arr[j+1].b)
{
if(arr[j].c==arr[j+1].c)
{
if(arr[j].d<arr[j+1].d)
{
continue;
}
else if(arr[j].d>arr[j+1].d)
{
temp=arr[j];
arr[j]=arr[j+1];
arr[j+1]=temp;
}
else if(arr[j].d==arr[j+1].d)
{
if(strcmp(arr[j].a,arr[j+1].a)>0)
{
temp=arr[j];
arr[j]=arr[j+1];
arr[j+1]=temp;
}
}
}
else if(arr[j].c<arr[j+1].c)
{
continue;
}
else if(arr[j].c>arr[j+1].c)
{
temp=arr[j+1];
arr[j+1]=arr[j];
arr[j]=temp;
}
}
}
}
for(i=1;i<=n;i++)
{
printf("%s\n",arr[i].a);
}
}
5 1 4 2 3
round1. a[1]에 와야하는 값 선택해서 교환 ( 최소값이 있는 자리를 찾기)
1 // 5 4 2 3
round2. a[2]에 와야 하는 값 선택해서 교환
1 2 // 4 5 3
round3.
1 2 3 // 5 4
round4.
1 2 3 4 // 5 -> end
*/
/*
#include <stdio.h>
int a[10001];
int n, i, j, temp, min;
int main() {
scanf("%d", &n);
for (i = 1; i <= n; i++)
scanf("%d", &a[i]);
for (i=1; i<n; i++) {
min=i;
for (j=i+1; j<=n; j++)
{
if(a[j]<a[min])
{
min=j;
}
}
temp = a[i];
a[i] = a[min];
a[min] = temp;
}
for (i=1; i<=n; i++)
printf("%d\n", a[i]);
return 0;
}
*/
/*
#include <stdio.h>
int a[101];
int i,j,n,temp,max;
int main()
{
scanf("%d",&n);
for(i=1;i<=n;i++)
{
scanf("%d",&a[i]);
}
for(i=1;i<n;i++)
{
max=i;
for(j=i+1;j<=n;j++)
{
if(a[j]>a[max])
{
max=j;
}
}
temp=a[i];
a[i]=a[max];
a[max]=temp;
}
for(i=1;i<=n;i++)
{
printf("%d ",a[i]);
}
return 0;
}
탐색 : 어떤 데이터셋에 내가 찾는 데이터가 있는지? 찾아보는것
방 50개
1. 순차탐색 1번방 2번방 3번방 .... 50번방
2. 이진탐색(이분탐색)-- ( 정렬이 되어있을 때만!!!)
1 ~50 사이의 수 1번 확인 -> 25개 -> 12 -> 6 -> 3 -> 1
start end
1 .......................n
k=3
1 3 5 7 9 10 11 13 15
1 2 3 4 5 6 7 8 9
#include <stdio.h>
int a[101];
int i,j,n,temp,max;
int bs(int start, int end, int k)
// a[start] ~ a[end] 에서 "k값의 위치" 리턴
{
if(a[end]<k) return -1; // 없으면
int mid = (start+end)/2;
if(a[mid]==k) return mid;
else if(k < a[mid]) return bs(start,mid-1,k);
else return bs(mid+1,end,k);
}
int main()
{
int k;
scanf("%d",&n);
for(i=1;i<=n;i++)
{
scanf("%d",&a[i]);
}
scanf("%d",&k);
printf("%d",bs(1,n,k));
}
*/
/*
#include <stdio.h>
int a[1000005]={};
int b[100001]={};
int main()
{
int i,j,n;
}
*/
0
0
2
wadepark2020
2024년 12월 29일
In 소스 코드 제출
/*
#include <stdio.h>
int main()
{
int a[50001]= {}, b[50001]= {};
int i,j,n,temp;
scanf("%d",&n);
for(i=1; i<=n; i++)
{
scanf("%d",&a[i]);
b[i] = a[i];
}
for(i=1; i<n; i++)
{
for(j=1; j<n; j++)
{
if(a[j]>a[j+1])
{
temp=a[j];
a[j]=a[j+1];
a[j+1]=temp;
}
}
}
for(i=1; i<=n; i++)
{
//b[i]가 a의 어디에 있는지?
for(j=1;j<=n;j++)
{
if(b[i]==a[j])
{
printf("%d ",j-1);
break;
}
}
}
return 0;
}
*/
/*
#include <stdio.h>
int main()
{
int a[101]={};
int i,j,n,temp;
for(i=1;i<=7;i++)
{
scanf("%d",&a[i]);
}
for(i=1;i<=7;i++)
{
for(j=1;j<=7;j++)
{
if(a[j]>a[j+1])
{
temp=a[j];
a[j]=a[j+1];
a[j+1]=temp;
}
}
}
printf("%d\n%d",a[j],a[j-1]);
return 0;
}
*/
/*
#include <stdio.h>
typedef struct
{
int a;
int b;
}myint;
int main()
{
myint arr[101];
myint temp;
int i,j,n;
scanf("%d",&n);
for(i=1;i<=n;i++)
{
scanf("%d %d",&arr[i].a,&arr[i].b);
}
for(i=1;i<n;i++)
{
for(j=1;j<=n-i;j++)
{
if(arr[j].a>arr[j+1].a)
{
temp=arr[j];
arr[j]=arr[j+1];
arr[j+1]=temp;
}
}
}
for(i=1;i<=n;i++)
{
printf("%d %d\n",arr[i].a,arr[i].b);
}
return 0;
}
*/
#include <stdio.h>
typedef struct
{
int a;
int b;
int c;
}myint;
int main()
{
int i,j,n;
myint temp;
myint arr[1001];
scanf("%d",&n);
for(i=1;i<=n;i++)
{
scanf("%d %d %d",&arr[i].a,&arr[i].b,&arr[i].c);
}
for(i=1;i<n;i++)
{
for(j=1;j<=n-i;j++)
{
if(arr[j].b>arr[j+1].b)
{
temp=arr[j];
arr[j]=arr[j+1];
arr[j+1]=temp;
}
else if(arr[j].b==arr[j+1].b)
{
if(arr[j].c>arr[j+1].c)
{
/////
}
}
}
}
for(i=1;i<=n;i++)
{
printf("%d %d %d",&arr[i].a,&arr[i].b,&arr[i].c);
}
return 0;
}
0
0
2
wadepark2020
2024년 12월 28일
In 소스 코드 제출
#include <stdio.h>
int main()
{
int a[50001]={}, b[50001]={};
int i,j,n,temp;
scanf("%d",&n);
for(i=1;i<=n;i++)
{
scanf("%d",&a[i]);
b[i] = a[i];
}
for(i=1;i<n;i++)
{
for(j=1;j<n;j++)
{
if(a[j]>a[j+1])
{
temp=a[j];
a[j]=a[j+1];
a[j+1]=temp;
}
}
}
for(i=0;i<=n;i++)
{
if(a[i])
{
printf("%d ",);
}
}
return 0;
}
0
0
4
wadepark2020
2024년 12월 22일
In 소스 코드 제출
/*
#include <stdio.h>
int main()
{
int a[50001]={}, b[50001]={};
int i,j,n,temp;
scanf("%d",&n);
for(i=1;i<=n;i++)
{
scanf("%d",&a[i]);
b[i] = a[i];
}
for(i=1;i<n;i++)
{
for(j=1;j<n;j++)
{
if(a[j]>a[j+1])
{
temp=a[j];
a[j]=a[j+1];
a[j+1]=temp;
}
}
}
for(i=0;i<=n;i++)
{
if(a[i])
{
printf("%d ",);
}
}
return 0;
}
*/
0
0
1
wadepark2020
2024년 12월 21일
In 소스 코드 제출
/*
#include <stdio.h>
int main()
{
int arr[100001]={};
int i,j,n,temp;
scanf("%d",&n);
for(i=1;i<=n;i++)
{
scanf("%d",&temp);
arr[temp]++;
}
for(i=0;i<=100000;i++)
{
for(j=0;j<arr[i];j++)
{
printf("%d ",i);
}
}
return 0;
}
*/
0
0
2
wadepark2020
2024년 12월 08일
In 소스 코드 제출
/*
#include <stdio.h>
int main()
{
int a[1001]={};
int i, j, n, temp, x=0;
scanf("%d",&n);
for(i=1;i<=n;i++)
{
scanf("%d",&a[i]);
}
for(i=1;i<=n;i++)
{
x=0;
for(j=1;j<=n-i;j++)
{
if(a[j]>a[j+1])
{
temp=a[j];
a[j]=a[j+1];
a[j+1]=temp;
x++;
}
}
if(x==0)
{
x=i-1;
break;
}
}
printf("%d",x);
return 0;
}
*/
/*
#include <stdio.h>
int main()
{
int a[4500005]={};
int i,j,n
scanf("%d",&n);
for(i=1;i<=n;i++)
{
scanf("%d",&a[i]);
}
for(i=1;i<=n;i++)
{
for(j=1;i<=n;j++)
{
}
}
for(i=1;i<=n;i++)
{
printf("%d",a[i]);
}
return 0;
}
*/
/*
temp=a[j];
a[j]=a[j+1];
a[j+1]=temp;
------------------->를 정렬을 안쓰고 바꾸기
*/
0
0
3
wadepark2020
2024년 12월 07일
In 소스 코드 제출
#include <stdio.h>
int main()
{
int a[1001]={};
int i,j,n,temp,sum=0,x=1;
scanf("%d",&n);
for(i=1;i<=n;i++)
{
scanf("%d",&a[i]);
}
for(i=1;i<=n;i++)
{
for(j=1;j<=n-i;j++)
{
if(a[j]>a[j+1])
{
temp=a[j];
a[j]=a[j+1];
a[j+1]=temp;
sum++;
}
}
if(sum==0)
{
x=i;
break;
}
}
printf("%d",x);
return 0;
}
0
0
3
wadepark2020
2024년 12월 01일
In 소스 코드 제출
/*
#include <stdio.h>
#include <string.h>
int front=-1;
int queue[205]={};
int back=-1;
void push(char x)
{
back++;
queue[back]=x;
}
void pop()
{
if(front<back)
{
front++;
}
}
void view()
{
printf("queue");
for(int i=0;i<=back;i++)
{
printf("%d ",queue[i]);
}
printf("back=%d\n",back);
}
int main()
{
int i,n,num;
char str[205]={};
scanf("%d",&n);
getchar();
for(i=0;i<n;i++)
{
scanf("%s",str);
if(str[1]=='u')
{
scanf("%d )",&num);
push(num);
}
else if(str[0]=='b')
{
if(back==front)
{
printf("-1");
printf("\n");
}
else
{
printf("%d\n",queue[back]);
}
}
else if(str[0]=='f')
{
if(back==front)
{
printf("-1");
printf("\n");
}
else
{
printf("%d\n",queue[front+1]);
}
}
else if(str[0]=='p')
{
pop();
}
else if(str[0]=='s')
{
printf("%d\n",back-front);//back은 게속 증가
}
else if(str[0]=='e')
{
if(back==front)
{
printf("true\n");
}
else
{
printf("false\n");
}
}
}
return 0;
}
정렬 (sort) : 정리해서 나열 ( 오름차순 , 내림차순 )
1. 코드 easy but 정렬속도 slow --- > (버블, 선택, 삽입)
2. 코드 hard but 정렬속도 fast --- > (퀵, 병합)
1. 버스 ( 만드는데 휩지만, 속도는 느려)
2. 비행기 (만드는데 어렵지만, 속도는 빨라)
( 항상 어느 정렬이 무조건 빠르다! 라고 할 수 없음 )
이름 특징
버블 항상 느려 , 코드가 엄청 간단
선택 중간 느려 , 코드가 "사람생각"
삽입 정렬이 어느정도 되어있는 경우 빠르다, 코드가 조금 복잡
퀵 데이터가 많을때 엄청 빨라, 코드가 복잡
1. 버블정렬 ( 제일 노답 성능 , 제일 간단 )
(옆에 있는 애랑 비교해서 바꿔 ) 를 걔속 반복
5 1 3 4 2 n=5
round1. (4번비교)
1 5 3 4 2
1 3 5 4 2
1 3 4 5 2
1 3 4 2 : 5
round2. (3번비교)
1 3 4 2 5
1 3 4 2 5
1 3 2 : 4 5
round3. ( 2번비교)
1 3 2 4 5
1 2 : 3 4 5
round4. ( 1번비교)
1 : 2 3 4 5
*/
/*
#include <stdio.h>
int a[10001];
int n, i, j, temp;
int main() {
scanf("%d", &n);
for (i=1; i<=n; i++)
scanf("%d", &a[i]);
for(i=1; i<n; i++)
{
for(j=1;j<=n-i;j++)
{
if (a[j] > a[j+1])
{
temp = a[j];
a[j] = a[j+1];
a[j+1] = temp;
}
}
}
for (i = 1; i <= n; i++)
printf("%d\n", a[i]);
return 0;
}
*/
/*
#include <stdio.h>
int main()
{
int i,j,n,temp;
int a[101]={};
scanf("%d",&n);
for(i=1;i<=n;i++)
{
scanf("%d",&a[i]);
}
for(i=1;i<n;i++)
{
for(j=1;j<=n-i;j++)
{
if(a[j]<a[j+1])
{
temp=a[j+1];
a[j+1]=a[j];
a[j]=temp;
}
}
}
for(i=1;i<=n;i++)
{
printf("%d ",a[i]);
}
return 0;
}
*/
/*
#include <stdio.h>
int main()
{
int a[1001]={};
int i,j,n,temp;
scanf("%d",&n);
for(i=1;i<=n;i++)
{
scanf("%d",&a[i]);
}
for(i=1;i<=n;i++)
{
for(j=1;j<=n-i;j++)
{
if(a[j]>a[j+1])
{
temp=a[j];
a[j]=a[j+1];
a[j+1]=temp;
}
}
}
}
*/
////////숙제:3011번 거품 정렬 풀어오기(좀 해와라)
0
0
3
wadepark2020
2024년 11월 30일
In 소스 코드 제출
/*
#include <stdio.h>
#include <string.h>
int top=0; //현재 놓인 막대 갯수
int sum=0; //현재 잘려진 조각 갯수
int main()
{
char a[100001]={};
int i;
scanf("%s",&a);
for(i=0;a[i]!=NULL;i++)
{
if(a[i]=='(')
{
if(a[i+1]=='(') //막대시작
{
top++;
}
else //레이저
{
sum+=top;
i++;
}
}
else if(a[i]==')') //막대끝
{
top--;
sum++;
}
}
printf("%d",sum);
return 0;
}
*/
/*
#include <stdio.h>
#include <string.h>
int front=0;
int queue[205]={};
int back=-1;
void push(char x)
{
back++;
queue[back]=x;
}
void pop()
{
if(front>back)
{
front++;
}
}
void view()
{
printf("queue");
for(int i=0;i<=back;i++)
{
printf("%d ",queue[i]);
}
printf("back=%d\n",back);
}
int main()
{
int i,n,cnt=0,num;
char str[205]={};
scanf("%d",&n);
getchar();
for(i=0;i<n;i++)
{
scanf("%s",str);
if(str[1]=='u')
{
scanf("%d )",&num);
push(num);
}
else if(str[0]=='t')
{
if(front==back)
{
printf("\n");
}
else
{
printf("%d\n",queue[back]);
}
}
}
if(str[0]=='p')
{
pop();
}
else if(str[0]=='s')
{
printf("%d\n",back+1);
}
else if(str[0]=='e')
{
if(front==back)
{
printf("true\n");
}
else
{
printf("false\n");
}
printf("\n");
}
return 0;
}
*/
0
0
1
wadepark2020
2024년 11월 30일
In 소스 코드 제출
#include <stdio.h>
#include <string,h>
char stack[100001]={};
int top=-1;
void push(char x)
{
top++;
stack[top]=x;
}
void pop()
{
top--;
}
int main()
{
char a[100001];
int i,sum=0,cnt=0;
scanf("%s",&a);
}
0
0
2
wadepark2020
2024년 11월 24일
In 소스 코드 제출
/*
#include <stdio.h>
#include <string.h>
int stack[205]={};
int top=-1; // top : 마지막 데이터의 "위치"
void push (char x)
{
top++;
stack[top]=x;
}
void pop()
{
if(top>-1)
{
top--;
}
}
void view()
{
printf(">>>>stack [");
for(int i=0;i<=top;i++)
{
printf("%d ",stack[i]);
}
printf("] top = %d \n",top);
}
int main()
{
int i,n,cnt=0, num;
char str[205]={};
scanf("%d",&n);
getchar();
for(i=0;i<n;i++)
{
scanf("%s",str);
// push? top? pop? size? empty?
if(str[1]=='u')
{
scanf("%d )",&num);
push(num);
}
else if(str[0]=='t')
{
if(top==-1)
{
printf("-1\n");
}
else
{
printf("%d\n",stack[top]);
}
}
else if(str[0]=='p')
{
pop();
}
else if(str[0]=='s')
{
printf("%d\n",top+1);
}
else if(str[0]=='e')
{
if(top==-1)
{
printf("true\n");
}
else
{
printf("false\n");
}
}
//view(); printf("\n");
}
return 0;
}
*/
/////////////////////////다시 해보기
#include <stdio.h>
#include <string.h>
int stacl[205{};
int top=-1;
void push(char x)
{
top++;
stack[top]=x;
}
void pop()
{
if(top>-1)
{
top--;
}
}
int main()
{
int i,n,cnt=0,num;
char str[205]={};
scanf("%d",&n);
for(i=0;i<=n-1;i++)
{
}
return 0;
}
0
0
4
wadepark2020
2024년 11월 10일
In 소스 코드 제출
/*
#include <stdio.h>
#include <string.h>
int main()
{
int i,x=0,y=0;
char str[100001]={};
scanf("%d %d",&x,&y);
gets(str);
for(i=0;str[i]!=NULL;i++)
{
if(str[i]=='(')
{
x=x+1;
}
else if(str[i]==')')
{
y=y+1;
}
}
printf("%d %d",x,y);
return 0;
}
*/
/*
#include <string.h>
#include <string.h>
char stack[50005]={};
int top=-1;
void push(char x)
{
top++;
stack[top]=x;
}
void pop()
{
top--;
}
int main()
{
int i;
char str[50005]={};
gets(str);
for(i=0;str[i]!=NULL;i++)
{
push(str[i]);
if(stack[top]==')' && top!=0)
{
if(stack[top-1]=='(')
{
pop();
pop();
}
else
{
printf("bad");
return 0;
}
}
}
if(top==-1)
{
printf("good");
}
else
{
printf("bad");
}
return 0;
}
// ')' 갯수 , '(' 갯수 세기
// if()//')'갯수 > '('갯수
// {
// printf("bad");
// return 0;
// }
*/
/*lude <stdio.h>
#include <string.h>
int stack[205]={};
int top=-1;
void push(int x)
{
top++;
stack[top]=x;
}
void pop()
{
top--;
}
int main()
{
int i,n,cnt=0;
char str[205]={};
scanf("%d",&n);
getchar();
for(i=0;i<n;i++)
{
gets(str);
// push? top? pop? size? empty?
if(str[1]=='u')
{
push(str[6]-48);
}
else if(str[0]=='t')
{
if(top==-1)
{
printf("-1\n");
}
else
{
printf("%d\n",stack[top]);
}
}
else if(str[0]=='p')
{
pop();
}
else if(str[0]=='s')
{
printf("%d\n",top+1);
}
else if(str[0]=='e')
{
if(top==-1)
{
printf("true\n");
}
else
{
printf("false\n");
}
}
}
return 0;//////////////숙제:틀린거 고쳐오기(오ㅓ답)
}
*/
0
0
4
wadepark2020
2024년 11월 03일
In 소스 코드 제출
/*
#include <stdio.h>
int stack[1005]={};
int top=-1;
void push(int b)
{
top++;
stack[top]=b;
}
void pop()
{
printf("%d ",stack [top]);
top--;
}
int main()
{
int i,a,n;
scanf("%d",&n);
for(i=1;i<=n;i++)
{
scanf("%d",&a);
push(a);
}
while(top!=-1)
{
pop(a);
}
return 0;
}*/
/*
#include <stdio.h>//////다시 한번 풀어보기
#include <string.h>
char stack[20]={};
int top=-1;
void push(char b)
{
top++;
stack[top]=b;
}
void pop()
{
printf("%c",stack[top]);
top--;
}
int main()
{
int i=0;
char a[20]={};
gets(a);
while(i<strlen(a))
{
push(a[i]);
i++;
}
while(top!=-1)
{
pop();
}
return 0;
}
*/
/*
#include <stdio.h>
#include <string.h>
char stack[20]={};
int top=-1;
void push(char x)
{
top++;
stack[top]=x;
}
void pop()
{
printf("%c",stack[top]);
top--;
}
int main()
{
int i=0;
char str[20]={};
gets(str);
for(i=0;str[i]!=NULL;i++)
{
//printf("%c",str[i]);
push(str[i]);
}
while(top!=-1)
{
pop();
}
}
8
12345678
12,345,678
*/
/*
#include <stdio.h>
#include <string.h>
char stack[305]={};
int top=-1;
void push(char x)
{
top++;
stack[top]=x;
}
void pop()
{
printf("%c",stack[top]);
top--;
}
int main()
{
int i,n;
char str[205]={};
scanf("%d\n",&n);
gets(str);
for(i=1;i<=n;i++)
{
if(i%3==1 && i!=1)
{
push(',');
}
push(str[n-i]);
}
while(top!=-1)
{
sum+=pop();
}
return 0;
}
*/
/*
#include <stdio.h>
#include <string.h>
char stack[20]={};
int top=-1;
void push(char x)
{
top++;
stack[top]=x;
}
void pop()
{
printf("%c",stack[top]);
top--;
}
int main()
{
int i=0;
char a[20]={};
gets(a);
while(i<strlen(a))
{
push(a[i]);
i++;
}
while(top!=-1)
{
pop();
}
return 0;
}*/
/*
#include<stdio.h>
int stack[100005]={};
int top=-1;
void push(int x)
{
top++;
stack[top]=x;
}
void pop()
{
top--;
}
int pop1()
{
pop();
return stack[top+1];
}*/
/*
int main()
{
int i,k,n,sum=0;
scanf("%d",&n);
for(i=1;i<=n;i++)
{
scanf("%d",&k);
if(k!=0)
{
push(k);
}
else if(k==0)
{
pop();
}
}
while(top!=-1)
{
sum+=pop1();
}
printf("%d",sum);
return 0;
}
*/
0
0
2
wadepark2020
2024년 10월 27일
In 소스 코드 제출
/*
#include <stdio.h> // 입출력문 printf, scanf 사용하기 위한 헤더
#include <windows.h> // move 사용하기위한 헤더
#include <conio.h> //_getch가 포함되어있는 헤더
#include <stdlib.h>
#include <time.h>
int pi=5, pj=5; // player의 위치
int ti,tj; // item $의 위치
int ai,aj; // 'a'ddress(item @의 위치)
int mi,mj; //마이너스의 m임!(item ?의 위치)
int ci,cj; //item &의 위치 // item &를 먹을경우, 조이스틱이 반대로 된다.
int ri,rj; //item #의 위치 // item #을 먹을 경우, 모든 아이템의 위치가 랜덤으로 바뀐다.
int si,sj; //item -의 위치// item -을 먹을 경우, 두칸씩 이동한다.
int hi,hj; //item !의 위치// item !을 먹을 경우, 1라운드로 돌아간다.
int speed=1;
int cnt=0;
int round=1;
void move(int i, int j)
{
COORD Pos;
Pos.X = j;
Pos.Y = i;
SetConsoleCursorPosition(GetStdHandle(STD_OUTPUT_HANDLE), Pos);
}
void map()
{
int i,j,cnt=0;
move(3,3);
for(i=3;i<=40;i++)
{
move(i,3);
for(j=3;j<=40;j++)
{
if(i==3 || j==3|| i==40 || j==40)
{
printf("*");
}
else
{
printf(" ");
}
}
}
}
void restart()
{
round=1;
cnt=0;
}
void init(){
system("cls");
//배경 출력
map();
//플레이어 출력
move(pi,pj);
printf("*");
// 아이템 랜덤 위치 정하고 출력
ti = rand()%36+4; // 4 ~ 39 사이의 랜덤수 생성
tj = rand()%36+4; // 4 ~ 39 사이의 랜덤수 생성
move(ti,tj);
printf("$");
ai=rand()%36+4;
aj=rand()%36+4;
move(ai,aj);
printf("@");
mi=rand()%36+4;
mj=rand()%36+4;
move(mi,mj);
printf("?");
ci=rand()%36+4;
cj=rand()%36+4;
move(ci,cj);
printf("&");//방향키가 반대로 되는 아이템이라서 다시 랜덤으로 위치 안나옴
// 현재 상태 나타내기
move(5,50);
printf("이걸 못해? 수준 ㅉ");
move(7,50);
printf("꼬우면 잘하던가 ㅋ 10점 찍으면 인정한다 ㅋ");
move(10,50);
printf(" coordinate(*의 좌표) : %2d %2d",pi,pj);
move(15,50);
printf(" score(점수) : %d",cnt);
move(25,50);
printf(" $(+1점) : %d %d",ti,tj); //아이템 $의 좌표 옆에 출력하기
move(30,50);
printf(" @(+2점) : %2d %2d",ai,aj); // 아이템 @의 좌표 옆에 출력하기
move(35,50);
printf(" ?(-3점) : %2d %2d",mi,mj); //아이템 ?의 좌표 옆에 출력하기
move(20,50);
printf(" &(방향키 반대로 ㅋ) : %d %d",ci,cj); //item&의 좌표 옆에 출력하기!!
if(round==2)
{
hi=rand()%36+4;
hj=rand()%36+4;
move(hi,hj);
printf("!");
si=rand()%36+4;
sj=rand()%36+4;
move(si,sj);
printf("-");
ri=rand()%36+4;
rj=rand()%36+4;
move(ri,rj);
printf("#");
move(35,50);
printf(" #(아이템들의 위치가 랜덤으로 초기화) :%d %d",ri,rj); //item#의 좌표 옆에 출력하기!!
move(45,50);
printf(" -(아이템을 먹엇을 때 두칸씩 이동) :%d %d",si,sj); //item-의 좌표 옆에 출력하기!!
move(47,50);
printf(" !(아이템을 먹엇을때 1라운드로 초기화): %d %d",hi,hj);
}
}
//i't'em=ti,tj
int main()
{
system("mode con:cols=110 lines=100");
int mode = 0; // 0 wasd위왼아오 , 1 sdwa위왼아오
int cnt=0; //점수
int ss=0;
srand(time(NULL));
// 플레이어 점수
init();
// 플레이어 이동 시작
for(;;)
{
// 키보드 입력받기
char x = _getch();
move(pi,pj);
printf(" ");
// 입력받은 문자에 따라 플레이어 위치 이동하기
if (mode==0) //좌우반전모드
{
if(x=='w')
{
if(pi-1*speed>3) pi=pi-1*speed;
}
else if(x=='a')
{
if(pj-1*speed>3)pj=pj-1*speed;
}
else if(x=='s')
{
if(pi+1*speed<40)pi=pi+1*speed;
}
else if(x=='d')
{
if(pj+1*speed<40)pj=pj+1*speed;
}
}
else
{
if(x=='s')
{
if(pi>=5) pi--;
}
else if(x=='d')
{
if(pj>=5)pj--;
}
else if(x=='w')
{
if(pi<=38)pi++;
}
else if(x=='a')
{
if(pj<=38)pj++;
}
}
// 이동된 위치에서 플레이어 출력하기
move(pi,pj);
printf("*");
if(pi==ti && pj==tj) // * item 먹었으면
{
cnt=cnt+1;
// 새로운 곳에 아이템을 생성
ti = rand()%36+4; // 4 ~ 39 사이의 랜덤수 생성
tj = rand()%36+4; // 4 ~ 39 사이의 랜덤수 생성
move(ti,tj);
printf("$");
}
else if(pi==ai && pj==aj)
{
cnt=cnt+2; //아이템을 먹었을때 2점을 획득
ai=rand()%36+4;
aj=rand()%36+4;
move(ai,aj);
printf("@");
}
else if(pi==mi && pj==mj)
{
cnt=cnt-3; //아이템을 먹었을때 3점 감점
if(cnt<3)
{
cnt=0;
}
mi=rand()%36+4;
mj=rand()%36+4;
move(mi,mj);
printf("?");
}
else if(pi==ci && pj==cj) // reverse 아이템
{
mode = 1;
}
else if(pi==ri && pj==rj) // 아이템 위치 바뀌는 아이템
{
move(ti,tj);
printf(" ");
ti = rand()%36+4; // 4 ~ 39 사이의 랜덤수 생성
tj = rand()%36+4; // 4 ~ 39 사이의 랜덤수 생성
move(ti,tj);
printf("$");
move(ai,aj);
printf(" ");
ai=rand()%36+4;
aj=rand()%36+4;
move(ai,aj);
printf("@");
move(mi,mj);
printf(" ");
mi=rand()%36+4;
mj=rand()%36+4;
move(mi,mj);
printf("?");
move(ci,cj);
printf(" ");
ci=rand()%36+4;
cj=rand()%36+4;
move(ci,cj);
printf("&");
ri=rand()%36+4;
rj=rand()%36+4;
move(ri,rj);
printf("#");
if(ss==0)
{
move(si,sj);
printf(" ");
si=rand()%36+4;
sj=rand()%36+4;
move(si,sj);
printf("-");
}
}
else if(pi==si && pj==sj)
{
speed=2;
ss=1;
}
else if(pi==hi && pj==hj)//1라운드로 초기화 !
{
restart();
init();
}
move(10,50);
printf(" coordinate(*의 좌표) : %2d %2d",pi,pj);
move(15,50);
printf(" score(점수) : %d",cnt);
move(25,50);
printf(" $(+1점) : %d %d",ti,tj); //아이템 $의 좌표 옆에 출력하기
move(30,50);
printf(" @(+2점) : %2d %2d",ai,aj); // 아이템 @의 좌표 옆에 출력하기
move(35,50);
printf(" ?(-3점) : %2d %2d",mi,mj); //아이템 ?의 좌표 옆에 출력하기
move(20,50);
printf(" &(방향키 반대로 ㅋ) : %d %d",ci,cj); //item&의 좌표 옆에 출력하기!!
if(round==2)
{
move(40,50);
printf(" &(방향키 반대로 ㅋ) : %d %d",ci,cj); //item&의 좌표 옆에 출력하기!!
move(35,50);
printf(" #(아이템들의 위치가 랜덤으로 초기화) :%d %d",ri,rj); //item#의 좌표 옆에 출력하기!!
move(45,50);
printf(" -(아이템을 먹엇을 때 두칸씩 이동) :%d %d",si,sj); //item-의 좌표 옆에 출력하기!!
move(47,50);
printf(" !(아이템을 먹엇을때 1라운드로 초기화): %d %d",hi,hj);
}
if(round==1 && cnt>=2)
{
round++;
system("cls");
move(20,20);
printf("1라운드 통과하셨습니다!!");/////////////////////////////////////////////////////////////////////////////////////////////////////////////////
move(21,20);
printf("스페이스 키를 누르시면 2라운드로 넘어갑니다");
char y=_getch();
if(y==' ') {
system("cls");
init();
continue;
}
}
}
}
자료구조 ->
자료 -> int a =10; a-> 자료
/*
#include <stdio.h>
int stack[1005]={};
int top=-1; // top : 마지막 데이터의 "위치" /// top==-1 -> stack이 비어있다
void push(int x)
{
top++;
stack[ top ] = x;
}
void pop()
{
printf("%d ",stack[top]);
top--;
}
int main()
{
int i, a, n;
scanf("%d",&n);
for(i=1;i<=n;i++)
{
scanf("%d",&a);
push(a); //a를 스택에 push
}
// stack에서 pop
//스택에 있는 값 모두 (보기)
// for(i=0;i<=top;i++)
// {
// printf("stack[%d] : %d\n",i,stack[i]);
// }
//스택에 있는 값 모두 (빼기 pop) + (보기)
while(top != -1)
{
printf("%d ",stack[top]);
top--;
}
return 0;
}
*/
#include <stdio.h>
int stack[1005]={};
int top=-1;
void push(int b)
{
top++;
stack[top]=b;
}
void pop()
{
printf("%d ",stack [top]);
top--;
}
int main()
{
int i,a,n;
scanf("%d",&n);
for(i=1;i<=n;i++)
{
scanf("%d",&a);
push(a);
}
while(top!=-1)
{
pop(a);
}
return 0;
}
*/
0
0
2
wadepark2020
2024년 10월 20일
In 소스 코드 제출
#include <stdio.h> // 입출력문 printf, scanf 사용하기 위한 헤더
#include <windows.h> // move 사용하기위한 헤더
#include <conio.h> //_getch가 포함되어있는 헤더
#include <stdlib.h>
#include <time.h>
void move(int i, int j)
{
COORD Pos;
Pos.X = j;
Pos.Y = i;
SetConsoleCursorPosition(GetStdHandle(STD_OUTPUT_HANDLE), Pos);
}
void map()
{
int i,j,cnt=0;
move(3,3);
for(i=3;i<=40;i++)
{
move(i,3);
for(j=3;j<=40;j++)
{
if(i==3 || j==3|| i==40 || j==40)
{
printf("*");
}
else
{
printf(" ");
}
}
}
}
//i't'em=ti,tj
int main()
{
system("mode con:cols=110 lines=100");
int mode = 0; // 0 wasd위왼아오 , 1 sdwa위왼아오
int pi=5, pj=5; // player의 위치
int ti,tj; // item $의 위치
int ai,aj; // 'a'ddress(item @의 위치)
int mi,mj; //마이너스의 m임!(item ?의 위치)
int ci,cj; //item &의 위치 // item &를 먹을경우, 조이스틱이 반대로 된다.
int ri,rj; //item #의 위치 // item #을 먹을 경우, 모든 아이템의 위치가 랜덤으로 바뀐다.
int si,sj; //item -의 위치// item -을 먹을 경우, 두칸씩 이동한다.
int hi,hj; //item !의 위치// item !을 먹을 경우, 1라운드로 돌아간다.
int speed=1;
srand(time(NULL));
// 플레이어 점수
int cnt=0;
//배경 출력
map();
//플레이어 출력
move(pi,pj);
printf("*");
// 아이템 랜덤 위치 정하고 출력
ti = rand()%36+4; // 4 ~ 39 사이의 랜덤수 생성
tj = rand()%36+4; // 4 ~ 39 사이의 랜덤수 생성
move(ti,tj);
printf("$");
ai=rand()%36+4;
aj=rand()%36+4;
move(ai,aj);
printf("@");
mi=rand()%36+4;
mj=rand()%36+4;
move(mi,mj);
printf("?");
ci=rand()%36+4;
cj=rand()%36+4;
move(ci,cj);
printf("&");
// 현재 상태 나타내기
move(5,50);
printf("이걸 못해? 수준 ㅉ");
move(7,50);
printf("꼬우면 잘하던가 ㅋ 10점 찍으면 인정한다 ㅋ");
move(10,50);
printf(" coordinate(*의 좌표) : %2d %2d",pi,pj);
move(20,50);
printf(" score(점수) : %d",cnt);
move(30,50);
printf(" $(+1점) : %d %d",ti,tj); //아이템 $의 좌표 옆에 출력하기
move(40,50);
printf(" @(+2점) : %2d %2d",ai,aj); // 아이템 @의 좌표 옆에 출력하기
move(45,50);
printf(" ?(-3점) : %2d %2d",mi,mj); //아이템 ?의 좌표 옆에 출력하기
move(25,50);
printf(" &(방향키 반대로 ㅋ) : %d %d",ci,cj); //item&의 좌표 옆에 출력하기!!
// 플레이어 이동 시작
for(;;)
{
// 키보드 입력받기
char x = _getch();
move(pi,pj);
printf(" ");
// 입력받은 문자에 따라 플레이어 위치 이동하기
if (mode==0)
{
if(x=='w')
{
if(pi>=5) pi--;
}
else if(x=='a')
{
if(pj>=5)pj--;
}
else if(x=='s')
{
if(pi<=38)pi++;
}
else if(x=='d')
{
if(pj<=38)pj++;
}
}
else
{
if(x=='s')
{
if(pi>=5) pi--;
}
else if(x=='d')
{
if(pj>=5)pj--;
}
else if(x=='w')
{
if(pi<=38)pi++;
}
else if(x=='a')
{
if(pj<=38)pj++;
}
}
// 이동된 위치에서 플레이어 출력하기
move(pi,pj);
printf("*");
if(pi==ti && pj==tj) // item 먹었으면
{
cnt=cnt+1;
// 새로운 곳에 아이템을 생성
ti = rand()%36+4; // 4 ~ 39 사이의 랜덤수 생성
tj = rand()%36+4; // 4 ~ 39 사이의 랜덤수 생성
move(ti,tj);
printf("$");
}
else if(pi==ai && pj==aj)
{
cnt=cnt+2; //아이템을 먹었을때 2점을 획득
ai=rand()%36+4;
aj=rand()%36+4;
move(ai,aj);
printf("@");
}
else if(pi==mi && pj==mj)
{
cnt=cnt-3; //아이템을 먹었을때 3점 감점
if(cnt<3)
{
cnt=0;
}
mi=rand()%36+4;
mj=rand()%36+4;
move(mi,mj);
printf("?");
}
else if(pi==ci && pj==cj)
{
mode = 1;
}
move(10,50);
printf(" coordinate(*의 좌표) : %2d %2d",pi,pj);
move(20,50);
printf(" score(점수) : %d",cnt);
move(30,50);
printf(" $(+1점) : %d %d",ti,tj); //아이템 $의 좌표 옆에 출력하기
move(40,50);
printf(" @(+2점) : %2d %2d",ai,aj); // 아이템 @의 좌표 옆에 출력하기
move(45,50);
printf(" ?(-3점) : %2d %2d",mi,mj); //아이템 ?의 좌표 옆에 출력하기
move(25,50);
printf(" &(방향키 반대로 ㅋ) : %d %d",ci,cj); //item&의 좌표 옆에 출력하기!!
if(cnt>=2)
{
break;
}
}
system("cls");
move(20,20);
printf("1라운드 통과하셨습니다!!");
move(21,20);
printf("스페이스 키를 누르시면 2라운드로 넘어갑니다");
char y=_getch();
if(y==' ')
{
int ss=0;
system("cls");
mode = 0; // 0 wasd위왼아오 , 1 sdwa위왼아오
pi=5, pj=5; // player의 위치
srand(time(NULL));
// 플레이어 점수
cnt=0;
//배경 출력
map();
//플레이어 출력
move(pi,pj);
printf("*");
// 아이템 랜덤 위치 정하고 출력
ti = rand()%36+4; // 4 ~ 39 사이의 랜덤수 생성
tj = rand()%36+4; // 4 ~ 39 사이의 랜덤수 생성
move(ti,tj);
printf("$");
ai=rand()%36+4;
aj=rand()%36+4;
move(ai,aj);
printf("@");
mi=rand()%36+4;
mj=rand()%36+4;
move(mi,mj);
printf("?");
ci=rand()%36+4;
cj=rand()%36+4;
move(ci,cj);
printf("&");
ri=rand()%36+4;
rj=rand()%36+4;
move(ri,rj);
printf("#");
si=rand()%36+4;
sj=rand()%36+4;
move(si,sj);
printf("-");
hi=rand()%36+4;
hj=rand()%36+4;
move(hi,hj);
printf("!");
// 현재 상태 나타내기
move(5,50);
printf("이걸 못해? 수준 ㅉ");
move(7,50);
printf("꼬우면 잘하던가 ㅋ 10점 찍으면 인정한다 ㅋ");
move(10,50);
printf(" coordinate(*의 좌표) : %2d %2d",pi,pj);
move(20,50);
printf(" score(점수) : %d",cnt);
move(30,50);
printf(" $(+1점) : %d %d",ti,tj); //아이템 $의 좌표 옆에 출력하기
move(40,50);
printf(" @(+2점) : %2d %2d",ai,aj); // 아이템 @의 좌표 옆에 출력하기
move(45,50);
printf(" ?(-3점) : %2d %2d",mi,mj); //아이템 ?의 좌표 옆에 출력하기
move(25,50);
printf(" &(방향키 반대로 ㅋ) : %d %d",ci,cj); //item&의 좌표 옆에 출력하기!!
move(47,50);
printf(" #(아이템들의 위치가 랜덤으로 초기화) :%d %d",ri,rj); //item#의 좌표 옆에 출력하기!!
move(49,50);
printf("-(아이템을 먹엇을 때 두칸씩 이동) :%d %d",si,sj); //item의 좌표 옆에 출력하기!!
move(46,50);
printf("!(아이템을 먹엇을때 1라운드로 초기화) : %d %d",hi,hj);//item의 좌표 옆에 출력하기!!
for(;;)
{
// 키보드 입력받기
char x = _getch();
move(pi,pj);
printf(" ");
// 입력받은 문자에 따라 플레이어 위치 이동하기
if (mode==0)
{
if(x=='w')
{
if(pi-1*speed>3) pi=pi-1*speed;
}
else if(x=='a')
{
if(pj-1*speed>3)pj=pj-1*speed;
}
else if(x=='s')
{
if(pi+1*speed<40)pi=pi+1*speed;
}
else if(x=='d')
{
if(pj+1*speed<40)pj=pj+1*speed;
}
}
// 이동된 위치에서 플레이어 출력하기
move(pi,pj);
printf("*");
if(pi==ti && pj==tj) // item 먹었으면
{
cnt=cnt+1;
// 새로운 곳에 아이템을 생성
ti = rand()%36+4; // 4 ~ 39 사이의 랜덤수 생성
tj = rand()%36+4; // 4 ~ 39 사이의 랜덤수 생성
move(ti,tj);
printf("$");
}
else if(pi==ai && pj==aj)
{
cnt=cnt+2; //아이템을 먹었을때 2점을 획득
ai=rand()%36+4;
aj=rand()%36+4;
move(ai,aj);
printf("@");
}
else if(pi==mi && pj==mj)
{
cnt=cnt-3; //아이템을 먹었을때 3점 감점
if(cnt<3)
{
cnt=0;
}
mi=rand()%36+4;
mj=rand()%36+4;
move(mi,mj);
printf("?");
}
else if(pi==ci && pj==cj)
{
mode = 1;
}
else if(pi==ri && pj==rj)
{
move(ti,tj);
printf(" ");
ti = rand()%36+4; // 4 ~ 39 사이의 랜덤수 생성
tj = rand()%36+4; // 4 ~ 39 사이의 랜덤수 생성
move(ti,tj);
printf("$");
move(ai,aj);
printf(" ");
ai=rand()%36+4;
aj=rand()%36+4;
move(ai,aj);
printf("@");
move(mi,mj);
printf(" ");
mi=rand()%36+4;
mj=rand()%36+4;
move(mi,mj);
printf("?");
move(ci,cj);
printf(" ");
ci=rand()%36+4;
cj=rand()%36+4;
move(ci,cj);
printf("&");
ri=rand()%36+4;
rj=rand()%36+4;
move(ri,rj);
printf("#");
move(si,sj);
if(ss==0)
{
printf(" ");
si=rand()%36+4;
sj=rand()%36+4;
move(si,sj);
printf("-");
}
}
else if(pi==si && pj==sj)
{
speed=2;
ss=1;
}
else if(pi==hi && pj==hj)
{
//1라운드로 초기화////////////////////////////////////////////////////////////////////////////////////////////////
}
move(10,50);
printf(" coordinate(*의 좌표) : %2d %2d",pi,pj);
move(20,50);
printf(" score(점수) : %d",cnt);
move(30,50);
printf(" $(+1점) : %d %d",ti,tj); //아이템 $의 좌표 옆에 출력하기
move(40,50);
printf(" @(+2점) : %2d %2d",ai,aj); // 아이템 @의 좌표 옆에 출력하기
move(45,50);
printf(" ?(-3점) : %2d %2d",mi,mj); //아이템 ?의 좌표 옆에 출력하기
move(25,50);
printf(" &(방향키 반대로 ㅋ) : %d %d",ci,cj); //item&의 좌표 옆에 출력하기!!
move(47,50);
printf(" #(아이템들의 위치가 랜덤으로 초기화) :%d %d",ri,rj); //item#의 좌표 옆에 출력하기!!
move(49,50);
printf(" -(아이템을 먹엇을 때 두칸씩 이동) :%d %d",si,sj); //item-의 좌표 옆에 출력하기!!
move(46,50);
printf(" !(아이템을 먹엇을 때 1라운드로 초기화):%d %d",hi,hj); //item!의 좌표 옆에 출력하기!!
if(cnt>=20)
{
break;
}
}
}
}
/*
#include <stdio.h>
int main()
{
srand(time(NULL));0
for(int i=0;i<10;i++)
{
//int x = rand(); // 0 ~ 324742 사이의 랜덤수
int x = rand()%10+1;
printf("%d ",x);
}
}*/
//숙제!!!!!!!!!!:문자들 수정, 좌표 설명해주는 설명창 예쁘게 정리!
0
0
3
wadepark2020
2024년 10월 20일
In 소스 코드 제출
#include <stdio.h> // 입출력문 printf, scanf 사용하기 위한 헤더
#include <windows.h> // move 사용하기위한 헤더
#include <conio.h> //_getch가 포함되어있는 헤더
#include <stdlib.h>
#include <time.h>
void move(int i, int j)
{
COORD Pos;
Pos.X = j;
Pos.Y = i;
SetConsoleCursorPosition(GetStdHandle(STD_OUTPUT_HANDLE), Pos);
}
void map()
{
int i,j,cnt=0;
move(3,3);
for(i=3;i<=40;i++)
{
move(i,3);
for(j=3;j<=40;j++)
{
if(i==3 || j==3|| i==40 || j==40)
{
printf("*");
}
else
{
printf(" ");
}
}
}
}
//i't'em=ti,tj
int main()
{
int mode = 0; // 0 wasd위왼아오 , 1 sdwa위왼아오
int pi=5, pj=5; // player의 위치
int ti,tj; // item $의 위치
int ai,aj; // 'a'ddress(item @의 위치)
int mi,mj; //마이너스의 m임!(item ?의 위치)
int ci,cj; //item &의 위치 // item &를 먹을경우, 조이스틱이 반대로 된다.
int ri,rj; //item #의 위치 // item #을 먹을 경우, 모든 아이템의 위치가 랜덤으로 바뀐다.
srand(time(NULL));
// 플레이어 점수
int cnt=0;
//배경 출력
map();
//플레이어 출력
move(pi,pj);
printf("*");
// 아이템 랜덤 위치 정하고 출력
ti = rand()%36+4; // 4 ~ 39 사이의 랜덤수 생성
tj = rand()%36+4; // 4 ~ 39 사이의 랜덤수 생성
move(ti,tj);
printf("$");
ai=rand()%36+4;
aj=rand()%36+4;
move(ai,aj);
printf("@");
mi=rand()%36+4;
mj=rand()%36+4;
move(mi,mj);
printf("?");
ci=rand()%36+4;
cj=rand()%36+4;
move(ci,cj);
printf("&");
// 현재 상태 나타내기
move(5,50);
printf("이걸 못해? 수준 ㅉ");
move(7,50);
printf("꼬우면 잘하던가 ㅋ 10점 찍으면 인정한다 ㅋ");
move(10,50);
printf(" coordinate(*의 좌표) : %2d %2d",pi,pj);
move(20,50);
printf(" score(점수) : %d",cnt);
move(30,50);
printf(" $(+1점) : %d %d",ti,tj); //아이템 $의 좌표 옆에 출력하기
move(40,50);
printf(" @(+2점) : %2d %2d",ai,aj); // 아이템 @의 좌표 옆에 출력하기
move(45,50);
printf(" ?(-3점) : %2d %2d",mi,mj); //아이템 ?의 좌표 옆에 출력하기
move(25,50);
printf(" &(방향키 반대로 ㅋ) : %d %d",ci,cj); //item&의 좌표 옆에 출력하기!!
// 플레이어 이동 시작
for(;;)
{
// 키보드 입력받기
char x = _getch();
move(pi,pj);
printf(" ");
// 입력받은 문자에 따라 플레이어 위치 이동하기
if (mode==0)
{
if(x=='w')
{
if(pi>=5) pi--;
}
else if(x=='a')
{
if(pj>=5)pj--;
}
else if(x=='s')
{
if(pi<=38)pi++;
}
else if(x=='d')
{
if(pj<=38)pj++;
}
}
else
{
if(x=='s')
{
if(pi>=5) pi--;
}
else if(x=='d')
{
if(pj>=5)pj--;
}
else if(x=='w')
{
if(pi<=38)pi++;
}
else if(x=='a')
{
if(pj<=38)pj++;
}
}
// 이동된 위치에서 플레이어 출력하기
move(pi,pj);
printf("*");
if(pi==ti && pj==tj) // item 먹었으면
{
cnt=cnt+1;
// 새로운 곳에 아이템을 생성
ti = rand()%36+4; // 4 ~ 39 사이의 랜덤수 생성
tj = rand()%36+4; // 4 ~ 39 사이의 랜덤수 생성
move(ti,tj);
printf("$");
}
else if(pi==ai && pj==aj)
{
cnt=cnt+2; //아이템을 먹었을때 2점을 획득
ai=rand()%36+4;
aj=rand()%36+4;
move(ai,aj);
printf("@");
}
else if(pi==mi && pj==mj)
{
cnt=cnt-3; //아이템을 먹었을때 3점 감점
if(cnt<3)
{
cnt=0;
}
mi=rand()%36+4;
mj=rand()%36+4;
move(mi,mj);
printf("?");
}
else if(pi==ci && pj==cj)
{
mode = 1;
}
move(10,50);
printf(" coordinate(*의 좌표) : %2d %2d",pi,pj);
move(20,50);
printf(" score(점수) : %d",cnt);
move(30,50);
printf(" $(+1점) : %d %d",ti,tj); //아이템 $의 좌표 옆에 출력하기
move(40,50);
printf(" @(+2점) : %2d %2d",ai,aj); // 아이템 @의 좌표 옆에 출력하기
move(45,50);
printf(" ?(-3점) : %2d %2d",mi,mj); //아이템 ?의 좌표 옆에 출력하기
move(25,50);
printf(" &(방향키 반대로 ㅋ) : %d %d",ci,cj); //item&의 좌표 옆에 출력하기!!
if(cnt>=2)
{
break;
}
}
system("cls");
move(20,20);
printf("1라운드 통과하셨습니다!!");
move(21,20);
printf("스페이스 키를 누르시면 2라운드로 넘어갑니다");
char y=_getch();
if(y==' ')
{
system("cls");
mode = 0; // 0 wasd위왼아오 , 1 sdwa위왼아오
pi=5, pj=5; // player의 위치
srand(time(NULL));
// 플레이어 점수
cnt=0;
//배경 출력
map();
//플레이어 출력
move(pi,pj);
printf("*");
// 아이템 랜덤 위치 정하고 출력
ti = rand()%36+4; // 4 ~ 39 사이의 랜덤수 생성
tj = rand()%36+4; // 4 ~ 39 사이의 랜덤수 생성
move(ti,tj);
printf("$");
ai=rand()%36+4;
aj=rand()%36+4;
move(ai,aj);
printf("@");
mi=rand()%36+4;
mj=rand()%36+4;
move(mi,mj);
printf("?");
ci=rand()%36+4;
cj=rand()%36+4;
move(ci,cj);
printf("&");
ri=rand()%36+4;
rj=rand()%36+4;
move(ri,rj);
printf("#");
// 현재 상태 나타내기
move(5,50);
printf("이걸 못해? 수준 ㅉ");
move(7,50);
printf("꼬우면 잘하던가 ㅋ 10점 찍으면 인정한다 ㅋ");
move(10,50);
printf(" coordinate(*의 좌표) : %2d %2d",pi,pj);
move(20,50);
printf(" score(점수) : %d",cnt);
move(30,50);
printf(" $(+1점) : %d %d",ti,tj); //아이템 $의 좌표 옆에 출력하기
move(40,50);
printf(" @(+2점) : %2d %2d",ai,aj); // 아이템 @의 좌표 옆에 출력하기
move(45,50);
printf(" ?(-3점) : %2d %2d",mi,mj); //아이템 ?의 좌표 옆에 출력하기
move(25,50);
printf(" &(방향키 반대로 ㅋ) : %d %d",ci,cj); //item&의 좌표 옆에 출력하기!!
move(47,50);
printf(" #(아이템들의 위치가 랜덤으로 초기화) :%d %d",ri,rj); //item#의 좌표 옆에 출력하기!!
for(;;)
{
// 키보드 입력받기
char x = _getch();
move(pi,pj);
printf(" ");
// 입력받은 문자에 따라 플레이어 위치 이동하기
if (mode==0)
{
if(x=='w')
{
if(pi>=5) pi--;
}
else if(x=='a')
{
if(pj>=5)pj--;
}
else if(x=='s')
{
if(pi<=38)pi++;
}
else if(x=='d')
{
if(pj<=38)pj++;
}
}
else
{
if(x=='s')
{
if(pi>=5) pi--;
}
else if(x=='d')
{
if(pj>=5)pj--;
}
else if(x=='w')
{
if(pi<=38)pi++;
}
else if(x=='a')
{
if(pj<=38)pj++;
}
}
// 이동된 위치에서 플레이어 출력하기
move(pi,pj);
printf("*");
if(pi==ti && pj==tj) // item 먹었으면
{
cnt=cnt+1;
// 새로운 곳에 아이템을 생성
ti = rand()%36+4; // 4 ~ 39 사이의 랜덤수 생성
tj = rand()%36+4; // 4 ~ 39 사이의 랜덤수 생성
move(ti,tj);
printf("$");
}
else if(pi==ai && pj==aj)
{
cnt=cnt+2; //아이템을 먹었을때 2점을 획득
ai=rand()%36+4;
aj=rand()%36+4;
move(ai,aj);
printf("@");
}
else if(pi==mi && pj==mj)
{
cnt=cnt-3; //아이템을 먹었을때 3점 감점
if(cnt<3)
{
cnt=0;
}
mi=rand()%36+4;
mj=rand()%36+4;
move(mi,mj);
printf("?");
}
else if(pi==ci && pj==cj)
{
mode = 1;
}
else if(pi==ri && pj==rj)
{
move(ti,tj);
printf(" ");
ti = rand()%36+4; // 4 ~ 39 사이의 랜덤수 생성
tj = rand()%36+4; // 4 ~ 39 사이의 랜덤수 생성
move(ti,tj);
printf("$");
move(ai,aj);
printf(" ");
ai=rand()%36+4;
aj=rand()%36+4;
move(ai,aj);
printf("@");
move(mi,mj);
printf(" ");
mi=rand()%36+4;
mj=rand()%36+4;
move(mi,mj);
printf("?");
move(ci,cj);
printf(" ");
ci=rand()%36+4;
cj=rand()%36+4;
move(ci,cj);
printf("&");
ri=rand()%36+4;
rj=rand()%36+4;
move(ri,rj);
printf("#");
}
move(10,50);
printf(" coordinate(*의 좌표) : %2d %2d",pi,pj);
move(20,50);
printf(" score(점수) : %d",cnt);
move(30,50);
printf(" $(+1점) : %d %d",ti,tj); //아이템 $의 좌표 옆에 출력하기
move(40,50);
printf(" @(+2점) : %2d %2d",ai,aj); // 아이템 @의 좌표 옆에 출력하기
move(45,50);
printf(" ?(-3점) : %2d %2d",mi,mj); //아이템 ?의 좌표 옆에 출력하기
move(25,50);
printf(" &(방향키 반대로 ㅋ) : %d %d",ci,cj); //item&의 좌표 옆에 출력하기!!
move(47,50);
printf(" #(아이템들의 위치가 랜덤으로 초기화) :%d %d",ri,rj); //item#의 좌표 옆에 출력하기!!
if(cnt>=20)
{
break;
}
}
}
}
// hw : 일정 점수가 넘어가면 라운드 상승 ( 어려워지도록, 두칸식움직여.. 감점 아이템 늘어나... 5번안에 못가면 아이템위치바껴 ,,,,,, )
//라운드와 라운드 사이에 ( 시각적인요소 라운드1 통과 라운드 2 시작 )
/*
#include <stdio.h>
int main()
{
srand(time(NULL));0
for(int i=0;i<10;i++)
{
//int x = rand(); // 0 ~ 324742 사이의 랜덤수
int x = rand()%10+1;
printf("%d ",x);
}
}*/
0
0
5
wadepark2020
2024년 10월 13일
In 소스 코드 제출
#include <stdio.h> // 입출력문 printf, scanf 사용하기 위한 헤더
#include <windows.h> // move 사용하기위한 헤더
#include <conio.h> //_getch가 포함되어있는 헤더
#include <stdlib.h>
#include <time.h>
void move(int i, int j)
{
COORD Pos;
Pos.X = j;
Pos.Y = i;
SetConsoleCursorPosition(GetStdHandle(STD_OUTPUT_HANDLE), Pos);
}
void map()
{
int i,j,cnt=0;
move(3,3);
for(i=3;i<=40;i++)
{
move(i,3);
for(j=3;j<=40;j++)
{
if(i==3 || j==3|| i==40 || j==40)
{
printf("*");
}
else
{
printf(" ");
}
}
}
}
//i't'em=ti,tj
int main()
{
int mode = 0; // 0 wasd위왼아오 , 1 sdwa위왼아오
int pi=5, pj=5; // player의 위치
int ti,tj; // item $의 위치
int ai,aj; // 'a'ddress(item @의 위치)
int mi,mj; //마이너스의 m임!(item ?의 위치)
int ci,cj; //item &의 위치 // item &를 먹을경우, 조이스틱이 반대로 된다.
srand(time(NULL));
// 플레이어 점수
int cnt=0;
//배경 출력
map();
//플레이어 출력
move(pi,pj);
printf("*");
// 아이템 랜덤 위치 정하고 출력
ti = rand()%36+4; // 4 ~ 39 사이의 랜덤수 생성
tj = rand()%36+4; // 4 ~ 39 사이의 랜덤수 생성
move(ti,tj);
printf("$");
ai=rand()%36+4;
aj=rand()%36+4;
move(ai,aj);
printf("@");
mi=rand()%36+4;
mj=rand()%36+4;
move(mi,mj);
printf("?");
ci=rand()%36+4;
cj=rand()%36+4;
move(ci,cj);
printf("&");
// 현재 상태 나타내기
move(50,10);
printf("이걸 못해? 수준 ㅉ");
move(55,5);
printf("꼬우면 잘하던가 ㅋ 30점 찍으면 인정한다 ㅋ");
move(10,50);
printf(" coordinate(*의 좌표) : %2d %2d",pi,pj);
move(20,50);
printf(" score(점수) : %d",cnt);
move(30,50);
printf(" $(+1점) : %d %d",ti,tj); //아이템 $의 좌표 옆에 출력하기
move(40,50);
printf(" @(+2점) : %2d %2d",ai,aj); // 아이템 @의 좌표 옆에 출력하기
move(50,50);
printf(" ?(-3점) : %2d %2d",mi,mj); //아이템 ?의 좌표 옆에 출력하기
move(60,50);
printf(" &(방향키 반대로 ㅋ) : %d %d",ci,cj); //item&의 좌표 옆에 출력하기!!
// 플레이어 이동 시작
for(;;)
{
// 키보드 입력받기
char x = _getch();
move(pi,pj);
printf(" ");
// 입력받은 문자에 따라 플레이어 위치 이동하기
if (mode==0)
{
if(x=='w')
{
if(pi>=5) pi--;
}
else if(x=='a')
{
if(pj>=5)pj--;
}
else if(x=='s')
{
if(pi<=38)pi++;
}
else if(x=='d')
{
if(pj<=38)pj++;
}
}
else
{
if(x=='s')
{
if(pi>=5) pi--;
}
else if(x=='d')
{
if(pj>=5)pj--;
}
else if(x=='w')
{
if(pi<=38)pi++;
}
else if(x=='a')
{
if(pj<=38)pj++;
}
}
// 이동된 위치에서 플레이어 출력하기
move(pi,pj);
printf("*");
if(pi==ti && pj==tj) // item 먹었으면
{
cnt=cnt+1;
// 새로운 곳에 아이템을 생성
ti = rand()%36+4; // 4 ~ 39 사이의 랜덤수 생성
tj = rand()%36+4; // 4 ~ 39 사이의 랜덤수 생성
move(ti,tj);
printf("$");
}
else if(pi==ai && pj==aj)
{
cnt=cnt+2; //아이템을 먹었을때 2점을 획득
ai=rand()%36+4;
aj=rand()%36+4;
move(ai,aj);
printf("@");
}
else if(pi==mi && pj==mj)
{
cnt=cnt-3; //아이템을 먹었을때 3점 감점
if(cnt<3)
{
cnt=0;
}
mi=rand()%36+4;
mj=rand()%36+4;
move(mi,mj);
printf("?");
}
else if(pi==ci && pj==cj)
{
mode = 1;
}
}
}
// hw : 일정 점수가 넘어가면 라운드 상승 ( 어려워지도록, 두칸식움직여.. 감점 아이템 늘어나... 5번안에 못가면 아이템위치바껴 ,,,,,, )
//라운드와 라운드 사이에 ( 시각적인요소 라운드1 통과 라운드 2 시작 )
/*
#include <stdio.h>
int main()
{
srand(time(NULL));0
for(int i=0;i<10;i++)
{
//int x = rand(); // 0 ~ 324742 사이의 랜덤수
int x = rand()%10+1;
printf("%d ",x);
}
}
*/
0
0
3
wadepark2020
2024년 10월 13일
In 소스 코드 제출
/*
#include <stdio.h> // 입출력문 printf, scanf 사용하기 위한 헤더
#include <windows.h> // move 사용하기위한 헤더
#include <conio.h> //_getch가 포함되어있는 헤더
#include <stdlib.h>
#include <time.h>
void move(int i, int j)
{
COORD Pos;
Pos.X = j;
Pos.Y = i;
SetConsoleCursorPosition(GetStdHandle(STD_OUTPUT_HANDLE), Pos);
}
void map()
{
int i,j,cnt=0;
move(3,3);
for(i=3;i<=40;i++)
{
move(i,3);
for(j=3;j<=40;j++)
{
if(i==3 || j==3|| i==40 || j==40)
{
printf("*");
}
else
{
printf(" ");
}
}
}
}
//i't'em=ti,tj
int main()
{
int mode = 0; // 0 wasd위왼아오 , 1 sdwa위왼아오
int pi=5, pj=5; // player의 위치
int ti,tj; // item $의 위치
int ai,aj; // 'a'ddress(item @의 위치)
int mi,mj; //마이너스의 m임!(item ?의 위치)
int ci,cj; //item &의 위치 // item &를 먹을경우, 조이스틱이 반대로 된다.
srand(time(NULL));
// 플레이어 점수
int cnt=0;
//배경 출력
map();
//플레이어 출력
move(pi,pj);
printf("*");
// 아이템 랜덤 위치 정하고 출력
ti = rand()%36+4; // 4 ~ 39 사이의 랜덤수 생성
tj = rand()%36+4; // 4 ~ 39 사이의 랜덤수 생성
move(ti,tj);
printf("$");
ai=rand()%36+4;
aj=rand()%36+4;
move(ai,aj);
printf("@");
mi=rand()%36+4;
mj=rand()%36+4;
move(mi,mj);
printf("?");
ci=rand()%36+4;
cj=rand()%36+4;
move(ci,cj);
printf("&");
// 현재 상태 나타내기
move(50,10);
printf("이걸 못해? 수준 ㅉ");
move(55,5);
printf("꼬우면 잘하던가 ㅋ 30점 찍으면 인정한다 ㅋ");
move(10,50);
printf(" coordinate(*의 좌표) : %2d %2d",pi,pj);
move(20,50);
printf(" score(점수) : %d",cnt);
move(30,50);
printf(" $(+1점) : %d %d",ti,tj); //아이템 $의 좌표 옆에 출력하기
move(40,50);
printf(" @(+2점) : %2d %2d",ai,aj); // 아이템 @의 좌표 옆에 출력하기
move(50,50);
printf(" ?(-3점) : %2d %2d",mi,mj); //아이템 ?의 좌표 옆에 출력하기
move(60,50);
printf(" &(방향키 반대로 ㅋ) : %d %d",ci,cj); //item&의 좌표 옆에 출력하기!!
// 플레이어 이동 시작
for(;;)
{
// 키보드 입력받기
char x = _getch();
move(pi,pj);
printf(" ");
// 입력받은 문자에 따라 플레이어 위치 이동하기
if (mode==0)
{
if(x=='w')
{
if(pi>=5) pi--;
}
else if(x=='a')
{
if(pj>=5)pj--;
}
else if(x=='s')
{
if(pi<=38)pi++;
}
else if(x=='d')
{
if(pj<=38)pj++;
}
}
else
{
if(x=='s')
{
if(pi>=5) pi--;
}
else if(x=='d')
{
if(pj>=5)pj--;
}
else if(x=='w')
{
if(pi<=38)pi++;
}
else if(x=='a')
{
if(pj<=38)pj++;
}
}
// 이동된 위치에서 플레이어 출력하기
move(pi,pj);
printf("*");
if(pi==ti && pj==tj) // item 먹었으면
{
cnt=cnt+1;
// 새로운 곳에 아이템을 생성
ti = rand()%36+4; // 4 ~ 39 사이의 랜덤수 생성
tj = rand()%36+4; // 4 ~ 39 사이의 랜덤수 생성
move(ti,tj);
printf("$");
}
else if(pi==ai && pj==aj)
{
cnt=cnt+2; //아이템을 먹었을때 2점을 획득
ai=rand()%36+4;
aj=rand()%36+4;
move(ai,aj);
printf("@");
}
else if(pi==mi && pj==mj)
{
cnt=cnt-3; //아이템을 먹었을때 3점 감점
if(cnt<3)
{
cnt=0;
}
mi=rand()%36+4;
mj=rand()%36+4;
move(mi,mj);
printf("?");
}
else if(pi==ci && pj==cj)
{
mode = 1;
}
}
}
/*
#include <stdio.h>
int main()
{
srand(time(NULL));
for(int i=0;i<10;i++)
{
//int x = rand(); // 0 ~ 324742 사이의 랜덤수
int x = rand()%10+1;
printf("%d ",x);
}
}
*/
0
0
1
wadepark2020
2024년 10월 13일
In 소스 코드 제출
#include <stdio.h> // 입출력문 printf, scanf 사용하기 위한 헤더
#include <windows.h> // move 사용하기위한 헤더
#include <conio.h> //_getch가 포함되어있는 헤더
#include <stdlib.h>
#include <time.h>
void move(int i, int j)
{
COORD Pos;
Pos.X = j;
Pos.Y = i;
SetConsoleCursorPosition(GetStdHandle(STD_OUTPUT_HANDLE), Pos);
}
void map()
{
int i,j,cnt=0;
move(3,3);
for(i=3;i<=40;i++)
{
move(i,3);
for(j=3;j<=40;j++)
{
if(i==3 || j==3|| i==40 || j==40)
{
printf("*");
}
else
{
printf(" ");
}
}
}
}
//i't'em=ti,tj
int main()
{
int pi=5, pj=5; // player의 위치
int ti,tj; // item 위치
srand(time(NULL));
// 플레이어 점수
int cnt=0;
//배경 출력
map();
//플레이어 출력
move(pi,pj);
printf("*");
// 아이템 랜덤 위치 정하고 출력
ti = rand()%36+4; // 4 ~ 39 사이의 랜덤수 생성
tj = rand()%36+4; // 4 ~ 39 사이의 랜덤수 생성
move(ti,tj);
printf("$");
// 현재 상태 나타내기
move(10,50);
printf(" coordinate : %2d %2d",pi,pj);
move(20,50);
printf(" score : %d",cnt);
move(30,50);
printf(" item : %d %d",ti,tj); //아이템의 좌표 옆에 출력하기
// 플레이어 이동 시작
for(;;)
{
// 키보드 입력받기
char x = _getch();
move(pi,pj);
printf(" ");
// 입력받은 문자에 따라 플레이어 위치 이동하기
if(x=='w')
{
if(pi>=5) pi--;
}
else if(x=='a')
{
if(pj>=5)pj--;
}
else if(x=='s')
{
if(pi<=38)pi++;
}
else if(x=='d')
{
if(pj<=38)pj++;
}
// 이동된 위치에서 플레이어 출력하기
move(pi,pj);
printf("*");
if(pi==ti && pj==tj) // item 먹었으면
{
cnt=cnt+1;
// 새로운 곳에 아이템을 생성
ti = rand()%36+4; // 4 ~ 39 사이의 랜덤수 생성
tj = rand()%36+4; // 4 ~ 39 사이의 랜덤수 생성
move(ti,tj);
printf("$");
}
// 현재 상태 나타내기
move(10,50);
printf(" coordinate : %2d %2d",pi,pj);
move(20,50);
printf(" score : %d",cnt); //아이템을 먹었을때의 점수 출력하기
move(30,50);
printf(" item : %d %d",ti,tj); //아이템의 좌표 풀력하기
}
}
/*
#include <stdio.h>
int main()
{
srand(time(NULL));
for(int i=0;i<10;i++)
{
//int x = rand(); // 0 ~ 324742 사이의 랜덤수
int x = rand()%10+1;
printf("%d ",x);
}
}
*/
0
0
8
wadepark2020
더보기
bottom of page