top of page
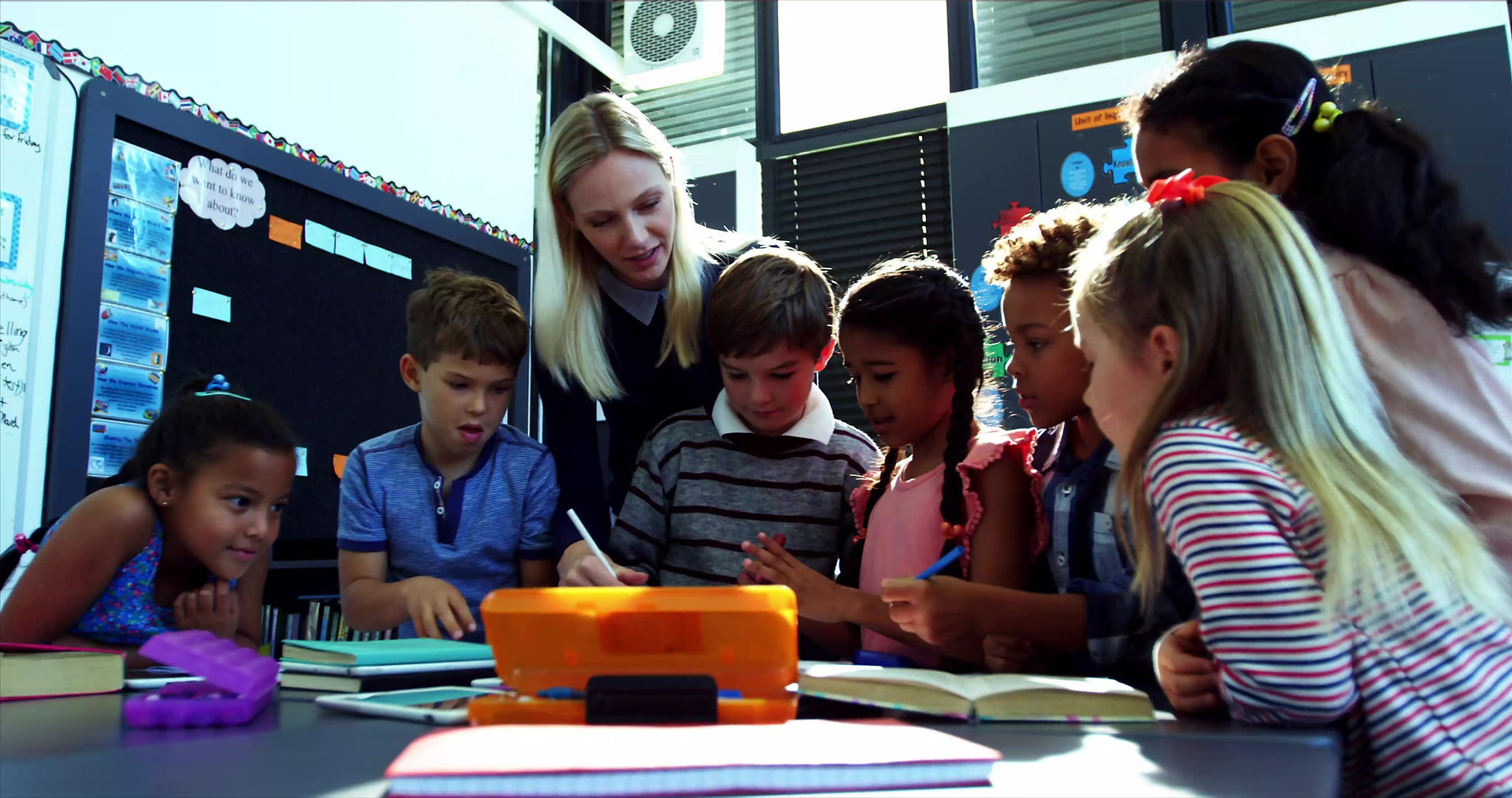
게시판 게시물
27woojaej
2024년 8월 04일
In 소스 코드 제출
/*
import java.util.*;
class student {
// 1. field
private String name;
int korScore, mathScore, engScore;
float mean;
int ranking;
//2. constructor
student() {
}
student(String name, int korScore, int mathScore, int engScore) {
this.name = name;
this.korScore = korScore;
this.mathScore = mathScore;
this.engScore = engScore;
this.mean = (float)((this.korScore + this.mathScore + this.engScore) / 3.0);
System.out.println(this.mean);
this.ranking=1;
}
// 3. method
String getName() {
return this.name;
}
void setName(String name) {
this.name=name;
}
}
public class Main {
public static void main(String[] args) {
Scanner t = new Scanner(System.in);
student []x = new student[5];
for(int i=0; i<5; i++) {
String name = t.next();
int kor = t.nextInt();
int math = t.nextInt();
int eng = t.nextInt();
x[i] = new student(name, kor, math, eng);
}
float max = -1;
float min = 2147483647;
String getName = "";
int rank = 1;
//x[3].name = "hi";
x[3].setName("hi");
//x[0] ~ x[4]
for(int i=0; i<x.length; i++) {
System.out.println(x[i].getName());
}
// System.out.println( getName.toString()+"is on the podium");
// descending sort ver1.
// for(int i=0; i<x.length; i++) {
// int max_index = 0;
// for(int j=0; j<x.length; j++) {
// if(x[max_index].mean < x[j].mean) {
// max_index=j;
// }
// }
// System.out.println(x[max_index].name + x[max_index].mean);
// x[max_index].mean=0;
// }
//descending sort ver2.
// for(int i=0; i<x.length; i++) {
// // x[i].mean의 랭킹 구하기
// for(int j=0; j<x.length; j++) {
// if(x[i].mean < x[j].mean) {
// x[i].ranking++;
// }
// }
// System.out.println(x[i].name+"은/는 "+x[i].ranking+"등 입네다");
// }
}
}
*/
//import java.util.*;
//class Person{
// String name;
// int age;
//
// public Person() {
// this.name = "name";
// this.age = 100;
// System.out.println("Person Constructer 1");
// }
// public Person(String name, int age) {
// System.out.println("Person Constructer 2");
// this.name = name;
// this.age = age;
// }
//
// void speak() {
// System.out.println("My name is "+ name+" and my age is "+age);
// }
//}
//
//// Class Inheritance ( super class, sub class)
//class Student extends Person{
// String school;
// int grade;
// public Student() {
// super("noname",100); // explicit call
// System.out.println("Student Constructer1");
// }
// public Student(String school, int grade) {
// super();
// System.out.println("Student Constructer2");
// }
//
// void speak() { //재정의 re-define overriding
// super.speak();
// System.out.println("hello");
// }
//
//}
//// 1. Teacher class 생성 ( + subject field)
//// 2. speak method overriding -> super.speak() + subject도 함께 소개하기
//class teacher extends Person{
// String subject;
// public teacher(String subject) {
//
// this.subject= "Individuals and Society";
// }
// void speak() {
// super.speak();
// System.out.println(subject);
// }
//}
//
//
////Object Oriented Programming -> 객체 지향 프로그래밍
//class Mid_Stu extends Student{
//
//}
//
//public class Main {
// public static void main(String[] args) {
// Student x = new Student("hello",7);
// x.speak();
//
// teacher y = new teacher("Individuals and Society");
// y.speak();
// }
//}
/*
class Circle {
private int radius;
public Circle(int radius) {
this.radius = radius;
}
public int getRadius() {
return radius;
}
}
class NamedCircle extends Circle {
String name;
public NamedCircle(int a, String name) {
super(a);
this.name = name;
}
void show() {
System.out.println(name+","+"반지름은"+getRadius());
}
}
public class Main {
public static void main(String[] args) {
NamedCircle w = new NamedCircle(5, "waffle");
w.show();
}
}
*/
//class point {
// private int x, y;
//
// public point(int x, int y) {
// this.x = x;
// this.y = y;
// }
// public int getX() {
// return x;
// }
// public int getY() {
// return y;
// }
// protected void move(int x, int y) {
// this.x = x;
// this.y = y;
// }
//}
//class Colorpoint extends point {
// String color;
// public Colorpoint (int x, int y, String color) {
// super(x, y);
// this.color = color;
// }
// void setpoint(int x, int y) {
// move(x, y);
// }
// void setColor(String color) {
// this.color = color;
// }
// void show() {
// System.out.println(color+"색으로"+"("+getX()+","+ getY()+")");
// }
//}
//public class Main {
// public static void main(String[] args) {
// Colorpoint cp = new Colorpoint(5, 5, "YELLOW");
// cp.setpoint(10, 20);
// cp.setColor("GREEN");
// cp.show();
//
// }
//}
//
//class Pen{
// private int amount;
//
// public int getAmount() {
// return amount;
// }
//
// public void setAmount(int amount) {
// this.amount = amount;
// }
//}
//class SharpPencil extends Pen{
// int width;
//}
//
//class BallPen extends Pen {
// private String color;
//
// public String getColor() {
// return color;
// }
//
// public void setColor(String color) {
// this.color = color;
// }
//}
//
//class FountainPen extends BallPen {
// public void refil(int n) {
// setAmount(n);
// }
//}
//class TV {
// private int size;
// public TV(int size) {
// this.size = size;
// }
// protected int getSize() {
// return size;
// }
//}
//class ColorTV extends TV {
// private int color;
// public ColorTV(int size,int color) {
// super(size);
// this.color = color;
// }
// protected int getColor() {
// return color;
// }
// void printproperty() {
// System.out.println(getSize()+"인치 "+color+"컬러");
// }
//}
//class IPTV extends ColorTV {
// private String IP;
// public IPTV(String IP, int size, int color) {
// super(size, color);
// this.IP = IP;
// }
// void printproperty() {
// System.out.println("나의 IPTV는 "+IP+" 주소의 "+ getSize()+"인치"+getColor()+"컬러");
// }
//}
//public class Main {
// public static void main(String[] args) {
// ColorTV myTV = new ColorTV(32, 1024);
// myTV.printproperty();
// IPTV iptv = new IPTV("192.1.1.2", 32, 2048);
// iptv.printproperty();
// }
//}
//
import java.util.Scanner;
abstract class Converter {
abstract protected double converter(double src);
abstract protected String getSrcString();
abstract protected String getDestString();
protected double ratio;
public void run() {
Scanner t = new Scanner(System.in);
System.out.println(getSrcString() + "을 " + getDestString() + "로 바꿉니다.");
System.out.println(getSrcString() + "을 입력하세요>> ");
double va1 = t.nextDouble();
double res = converter(va1);
System.out.println("변환 결과: " + res + getDestString() + "입니다");
t.close();
}
}
class Won2Dollar extends Converter {
public Won2Dollar(double ratio) {
this.ratio = ratio;
}
protected double converter(double src) {
return src/ratio;
}
protected String getSrcString() {
return "원";
}
protected String getDestString() {
return "달러";
}
}
public class Main {
public static void main(String[] args) {
Won2Dollar toDollar = new Won2Dollar(1200);
toDollar.run();
}
}
0
0
6
27woojaej
2024년 8월 03일
In 소스 코드 제출
import java.util.*;
class student {
String name;
int korScore, mathScore, engScore;
float mean;
int ranking;
student() {
}
student(String name, int korScore, int mathScore, int engScore) {
this.name = name;
this.korScore = korScore;
this.mathScore = mathScore;
this.engScore = engScore;
this.mean = (float)((this.korScore + this.mathScore + this.engScore) / 3.0);
System.out.println(this.mean);
this.ranking=0;
}
}
public class Main {
public static void main(String[] args) {
Scanner t = new Scanner(System.in);
student []x = new student[5];
for(int i=0; i<5; i++) {
String name = t.next();
int kor = t.nextInt();
int math = t.nextInt();
int eng = t.nextInt();
x[i] = new student(name, kor, math, eng);
}
float max = -1;
float min = 2147483647;
String getName = "";
int rank = 0;
for(int i=0; i<x.length; i++) {
if(x[i].mean > max) {
getName = x[i].name;
max = x[i].mean;
}
}
// System.out.println( getName.toString()+"is on the podium");
for(int i=0; i<x.length; i++) {
for(int j=0; j<x.length; j++) {
if(x[i].mean>x[j].mean&&j!=i) {
rank= x[i].ranking;
getName=x[i].name;
}
}
x[i].ranking+=1;
System.out.println(rank);
System.out.println(getName);
}
}
}
0
0
5
27woojaej
2024년 8월 02일
In 소스 코드 제출
import java.util.*;
class student {
int max=0;
int[] arr=new int[5];
String name;
int kor, math, eng;
student() {
}
student(String name, int kor, int math, int eng) {
this.name=name;
this.kor=kor;
this.math=math;
this.eng=eng;
}
void mean() {
int mean=(kor+math+eng)/3;
System.out.println(name+"의 "+"평균은 " + mean);
}
}
public class Main {
public static void main(String[] args) {
String honour;
int max=0;
Scanner t = new Scanner(System.in);
// 클래스의 배열 선언: 주소값 할당만을 위한 준비
student []x = new student[5];
for(int i=0; i<x.length; i++) {
x[i] = new student();
x[i].name = t.next();
x[i].kor = t.nextInt();
x[i].math = t.nextInt();
x[i].eng = t.nextInt();
x[i].mean();
}
for(int i=0;i<x.length;i++) {
if(mean>max) {
max=mean;
honour = x[i].name;
}
System.out.println(honour);
}
}
}
0
1
11
27woojaej
2024년 7월 31일
In 소스 코드 제출
함수 function (기능) : 심부름
명령의 묶음 : 중복을 줄이고, 효율적으로 코드 작성
method 메소드
*/
//import java.util.*;
//public class Main {
//
//// public static void f(int money){
//// System.out.println(money+"원을 받았습니다");
//// System.out.println("학원을 나가기");
//// System.out.println("양꼬치사먹기");
//// System.out.println("학원으로 돌아오기");
//// }
// public static String f(int money,int count){
// System.out.println(money+"원을 받았습니다"+count+"개 사오기");
// System.out.println("학원을 나가기");
// System.out.println("양꼬치사서챙기기");
// System.out.println("학원으로 돌아오기");
// return "양꼬치";
// }
//
//
// public static void main(String[] args) {
//
// System.out.println(f(50000,50));
//
// }
//
//}
//public class Main {
// public static void f() {
// System.out.println("hello");
// }
// public static void main(String[] args) {
// f();
// }
//}
//public class Main {
// public static void f() {
// System.out.println("A");
// }
// public static void main(String[] args) {
// f();
// }
//}
//import java.util.*;
//public class Main {
// public static void f(int a) {
// if(a==1) {
// System.out.println("hello");
// }
// if(a==2) {
// System.out.println("world");
// }
// }
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int a = t.nextInt();
// f(a);
// }
//}
//import java.util.*;
//public class Main {
// public static void f(int a) {
// long ans = 0;
// for(int i=1;i<=a;i++) {
// ans+=i;
// }
// System.out.println(ans);
// }
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int a = t.nextInt();
// f(a);
// }
//}
//import java.util.*;
//public class Main {
// public static void f(int n) {
// long ans=1;
// for(int i=n;i>=1;i--) {
// ans*=i;
// }
// System.out.println(ans);
// }
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int n = t.nextInt();
// f(n);
// }
//}
//import java.util.*;
//public class Main {
// public static void f(int a) {
// int yaksu=0;
// for(int i=1;i<=a;i++) {
// if(a%i==0) {
// yaksu++;
// }
// }
// if(yaksu==2) {
// System.out.println("prime");
// }
// else if(yaksu>=3) {
// System.out.println("composite");
// }
// }
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int a=t.nextInt();
// f(a);
// }
//}
//import java.util.*;
//public class Main {
// public static char grade(int a) {
// if(a>=90) {
// return 'A';
// }
// else if(a>=80&&a<90) {
// return 'B';
// }
// else if(a>=70&&a<80) {
// return 'C';
// }
// else if(a>=60&&a<70) {
// return 'D';
// }
// else {
// return 'F';
// }
// }
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int a = t.nextInt();
// System.out.print(grade(a));
// }
//}
//import java.util.*;
//public class Main {
// public static int f(int a) {
// int yaksu=0;
// for(int i=1;i<=a;i++) {
// if(a%i==0) {
// yaksu++;
// }
// }
// return yaksu;
// }
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int a = t.nextInt();
// System.out.println(f(a));
// }
//}
//import java.util.*;
//public class Main {
// public static long f(long a) {
// long ans=0;
// for(;;) {
// long asdf = a%10;
// ans = ans*10+asdf;
// a = a/10;
// if(a==0) {
// break;
// }
// }
// return ans;
// }
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// long a= t.nextLong();
// System.out.println(f(a));
// }
//}
//import java.util.*;
//public class Main {
// public static void f(int a) {
// System.out.printf("%.2f",a*a*3.14);
// }
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int a = t.nextInt();
// f(a);
// }
//}
import java.util.*;
public class Main {
public static int f(int a) {
int ans=0;
for(;;) {
int asdf = a%10;
ans = ans+asdf;
a = a/10;
if(a==0) {
break;
}
}
return ans;
}
public static void main(String[] args) {
int b=0;
Scanner t=new Scanner(System.in);
int a=t.nextInt();
b = f(a);
while(b>9) {
b = f(b);
}
System.out.println(b);
}
}
0
0
2
27woojaej
2024년 7월 26일
In 소스 코드 제출
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int n = t.nextInt();
// int[] arr= new int[n];
// for(int i=0;i<n;i++) {
// arr[i]=t.nextInt();
// }
// for(int i=0;i<n;i++) {
// System.out.print(i+1+": ");
// for(int j=0;j<n;j++) {
// if(arr[j]>arr[i]&&j!=i) {
// System.out.print("< ");
// }
// else if(arr[j]==arr[i]&&j!=i) {
// System.out.print("= ");
// }
// else if(arr[j]<arr[i]&&j!=i){
// System.out.print("> ");
// }
// }
// System.out.println();
// }
// }
//}
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// String x = t.nextLine();
// for(int i=0; i<x.length(); i++) {
// if(x.charAt(i)!=' ') {
// System.out.print(x.charAt(i));
// }
// }
// }
//}
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int n = t.nextInt();
// int sum = 0;
// int max = 0;
// int[] arr=new int[n];
// for(int i=0;i<n-1;i++) {
// arr[i]=t.nextInt();
// sum+=arr[i];
// }
// for(int j=1;j<=n;j++) {
// max+=j;
// }
// System.out.println(max-sum);
// }
//}
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// String x = t.nextLine();
// for(int i='a';i<='z';i++) {
// System.out.println((char)i+":");
// if(x.charAt(i)==(char)i) {
// i+=1;
// }
// }
// }
//}
//public class Main {
// public static void main(String[] args) {
// for(int i=0; i<26; i++) {
// System.out.println((char)(i+'A'));
// }
// }
//}
import java.util.*;
public class Main {
public static void main(String[] args) {
Scanner t = new Scanner(System.in);
String a = t.nextLine();
int somunja = 0;
int daemunja = 0;
for(int i=0;i<a.length();i++) {
if(a.charAt(i)>='a'&&a.charAt(i)<='z') {
somunja++;
}
}
for(int j=0;j<a.length();j++) {
if(a.charAt(j)>='A'&&a.charAt(j)<='Z') {
daemunja++;
}
}
System.out.print("소문자의 갯수"+":"+somunja+"\n");
System.out.print("대문자의 갯수"+":"+daemunja);
}
}
0
0
5
27woojaej
2024년 7월 24일
In 소스 코드 제출
import java.util.Scanner;
/*
* 1차원 선
* 2차원 면
* 3차원 입체
*
* 1차원 배열(array)
* 선 형태로 나열한 것
*/
/*
import java.util.*;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
// 정수 변수 5개 관리
//int a, b, c, d, e;
// 정수 변수 100개 관리 (입력, 출력, 찾기 ..)
int[] arr; // 이름 짓기
arr = new int[100]; // 100개의 집 짓기
//arr[0] arr[1] arr[2] ... arr[99]
int[] arr1 = new int[100]; //이름지으면서 집도짓기
System.out.println("만들 배열의 갯수를 입력하세욧>>");
int n = sc.nextInt();
int[] house = new int[n]; //n개의 배열 만들기
System.out.println("house[3]에 넣을 정수를 입력하세요>>");
house[3] = sc.nextInt();
System.out.println("배열의 길이 " + house.length);
for(int i=0;i<house.length ; i++) {
System.out.println("house["+i+"] = "+house[i]);
}
float[] arr3 = new float[100];
arr3[0] = sc.nextFloat();
System.out.println(arr3[0]);
}
}
*/
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int [] arr=new int[5];
// for(int i=0;i<5;i++) {
// arr[i] = t.nextInt();
// }
// for(int c=4;c>=0;c--) {
// System.out.print(arr[c]+" ");
// }
// }
//}
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int a = t.nextInt();
// int [] arr=new int[a];
// for(int i=0;i<a;i++) {
// arr[i] = t.nextInt();
// }
// for(int x=a-1;x>=0;x--) {
// System.out.print(arr[x]+" ");
// }
// }
//}
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int [] arr=new int[10];
// int av = 0;
// float average = 0;
// int s = 0;
// int b = 0;
// for(int i=0;i<10;i++) {
// arr[i] = t.nextInt();
// av += arr[i];
// }
// average = (float)av/10;
// System.out.println(average);
// for(int i=0;i<10;i++) {
// if(arr[i]<average) {
// s += 1;
//
// }
// else {
// b += 1;
// }
// }
// System.out.println(b+" "+s);
// }
//}
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int a = t.nextInt();
// int [] arr=new int[a];
// for(int i=0;i<a;i++) {
// arr[i]= t.nextInt();
// }
// for(int x=1;x<=2;x++) {
// for(int i=0;i<a;i++) {
// System.out.println(arr[i]);
// }
// }
// }
//}
/*
1 2 3 4 5
2 3 4 5
3 4 5
4 5
5
*/
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int x = t.nextInt();
// int [] arr= new int[x];
// for(int i=0;i<x;i++) {
// arr[i]=t.nextInt();
// }
// for(int i=0;i<x;i++) {
// for(int j=i;j<x;j++) {
// System.out.print(arr[j]+" ");
// }
// for(int y=0;y<i;y++) {
// System.out.print(arr[y]+" ");
// }
// System.out.println();
// }
// }
//}
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int [] arr= new int[10];
// for(int i=0;i<10;i++) {
// arr[i]=t.nextInt();
// }
// int k = t.nextInt();
// System.out.print(arr[k-1]);
// }
//}
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int n = t.nextInt();
// int k = 0;
// int[] arr=new int[24];
// for(int i=0;i<n;i++) {
// k=t.nextInt();
// arr[k]++;
// }
// for(int i=1;i<=23;i++) {
// System.out.print(arr[i]+" ");
// }
// }
//}
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int a = t.nextInt();
// int [] arr= new int[a];
// int min = 10000;
// for(int i=0;i<a;i++) {
// arr[i]=t.nextInt();
// }
// for(int i=0;i<a;i++) {
// if(arr[i]<min) {
// min=arr[i];
// }
// }
// System.out.print(min);
// }
//}
import java.util.*;
public class Main {
public static void main(String[] args) {
Scanner t = new Scanner(System.in);
int
}
}
0
0
2
27woojaej
2024년 7월 19일
In 소스 코드 제출
/import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int x = t.nextInt();
// for (int i=1;i<=x;i++) {
// for(int j=1;j<=x;j++) {
// if(i==1||i==x) {
// System.out.print("*");
// }
// else if(j==1||j==x) {
// System.out.print("*");
// }
// else {
// System.out.print(" ");
// }
// }
// System.out.println();
// }
// }
//}
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int a = t.nextInt();
// for (int i=1;i<=a;i++) {
// for (int j=1;j<=a;j++) {
// if(i==1||i==a) {
// System.out.print("*");
// }
// else if(j==1||j==a) {
// System.out.print("*");
// }
// else if (j+i==a+1) {
// System.out.print("*");
// }
// else if(i-j==0) {
// System.out.print("*");
// }
// else {
// System.out.print(" ");
// }
// }
// System.out.println();
// }
// }
//}
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int l = t.nextInt();
// for(int i=1;i<=l;i++) {
// for(int j=1;j<=l;j++) {
// if(i==1||i==l) {
// System.out.print("*");
// }
// else if(j==1||j==l) {
// System.out.print("*");
// }
// else if(j+i==l+1) {
// System.out.print("*");
// }
// else if(i-j==0) {
// System.out.print("*");
// }
// else if(j==l/2+1) {
// System.out.print("*");
// }
// else if((l+1)/2==i) {
// System.out.print("*");
// }
// else {
// System.out.print(" ");
// }
// }
// System.out.println();
// }
// }
//}
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int a = t.nextInt();
//
// for(int i=0; i<=a/2; i++) {
// for(int j=i; j<a/2; j++) {
// System.out.print(" ");
// }
// for(int j=0; j<=i*2; j++) {
// System.out.print("*");
// }
// System.out.println();
// }
// }
//}
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int a = t.nextInt();
// int b = t.nextInt();
// for(int x=1;x<=b;x++) {
// for(int i=1;i<=a;i++) {
// for(int j=2;j<=i;j++) {
// System.out.print(" ");
// }
// System.out.println("*");
// }
// for(int i=a;i-1>=1;i--) {
// for(int j=2;j<=i-1;j++) {
// System.out.print(" ");
// }
// System.out.println("*");
// }
// }
// }
//}
//import java.util.*;
//
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int arr[] = new int[100];
// // arr[0], arr[1], arr[2], .., arr[98], arr[99]
//
// for(int i=0; i<arr.length; i++) {
// arr[i] = t.nextInt();
// }
//
// for(int i=0; i<arr.length; i++) {
// System.out.println(arr[i]);
// }
//
//
//
//
//
//
// }
//}
import java.util.*;
public class Main {
public static void main(String[] args) {
Scanner t = new Scanner(System.in);
int a = t.nextInt();
for(int i=1;i<=a;i++) {
for(int j=1;j<=a;j++) {
System.out.print(".");
}
System.out.println();
for(int j=1;j<=i;j++) {
System.out.print("*");
}
System.out.println();
}
}
}
0
0
3
27woojaej
2024년 7월 17일
In 소스 코드 제출
import java.util.*;
public class Main {
public static void main(String[] args) {
Scanner t = new Scanner(System.in);
int a = t.nextInt();
// for(int i=1;i<=a;i++) {
// for(int j=1;j<=i;j++) {
// System.out.print("*");
// }
// System.out.println();
// }
for(int i=a;i>=1;i--) {
for(int j=1;j<=i-1;j++) {
System.out.print(" ");
}
for(int j=1;j<=a;j++) {
System.out.print("*");
}
System.out.println();
}
}
}
*/
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int a = t.nextInt();
// for(int i=a;i>=1;i--) {
// for(int j=1;j<=i-1;j++) {
// System.out.print(" ");
// }
// for(int j=1;j<=a;j++) {
// System.out.print("*");
// }
// System.out.println();
// }
// }
//}
import java.util.*;
public class Main {
public static void main(String[] args) {
Scanner t = new Scanner(System.in);
String[] arr = t.nextLine().split(" ");
int h = Integer.valueOf(arr[0]);
int k = Integer.valueOf(arr[1]);
char d = arr[2].charAt(0);
if(d=='R') {
for(int i=h;i>=1;i--) {
for(int j=1;j<=i-1;j++) {
System.out.print(" ");
}
for(int j=1;j<=k;j++) {
System.out.print("*");
}
System.out.println();
}
}
else if(d=='L') {
for(int i=1;i<=h;i++) {
for(int j=1;j<=i-1;j++) {
System.out.print(" ");
}
for(int j=1;j<=k;j++) {
System.out.print("*");
}
System.out.println();
}
}
}
}
0
0
1
27woojaej
2024년 7월 12일
In 소스 코드 제출
////import java.util.*;
//// public class Main {
//// public static void main(String[] args) {
//// Scanner t = new Scanner(System.in);
//// int a = t.nextInt();
//// int b = t.nextInt();
//// if (a<=b) {
//// int sum = 0;
//// for (int i=a; i<=b;i++) {
//// if (i%2==1) {
//// sum = sum+i;
//// System.out.print("+"+i);
//// }
//// else {
//// sum = sum-i;
//// System.out.print("-"+i);
//// }
//// }
//// System.out.print("="+sum);
//// }
//// }
////}
////import java.util.*;
////public class Main {
//// public static void main(String[] args) {
//// Scanner sc = new Scanner(System.in);
//// int n = sc.nextInt();
//// for (int i=1;;i++) {
//// if((i*i)>=n) {
//// System.out.println((n-(i-1)*(i-1))+" "+(i-1));
//// break;
//// }
//// }
//// }
////}
////import java.util.*;
////public class Main {
//// public static void main(String[] args) {
//// Scanner t = new Scanner(System.in);
//// int a = t.nextInt();
//// int d = t.nextInt();
//// int n = t.nextInt();
//// for(int i=0; i<n-1; i++) {
//// a += d;
//// }
//// System.out.println(a);
//// }
////}
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int a = t.nextInt();
// int r = t.nextInt();
// int n = t.nextInt();
// for(int i=0; i<=n; i++) {
// a += a+r*i;
// }
// System.out.println(a);
// }
// }
//
//
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int a = 9;
// int max = 0;
// int location = 0;
// for (int i=1; i<=a;i++) {
// int b = t.nextInt();
// if (max<b) {
// max=b;
// location = i;
// }
// }
// System.out.println(max);
// System.out.println(location);
// }
//}
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int a = 5;
// int min = 1000000;
// int max = -1000000;
// for(int i=1;i<=a;i++) {
// int n = t.nextInt();
// if (max<n) {
// max=n;
// }
// if (min>n) {
// min=n;
// }
// }
// System.out.println(max);
// System.out.println(min);
// }
//}
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int a = 7;
// int sum = 0;
// int f = 0;
// int min = 100;
// for(int i=1;i<=a;i++) {
// int n = t.nextInt();
// if(n%2!=0) {
// f = 1;
// sum += n;
// if(min>n) {
// min=n;
// }
// }
// }
// if (f == 0) {
// System.out.println("-1");
// }
// else {
// System.out.println(sum);
// System.out.println(min);
// }
// }
//}
//import java.util.*;
//public class Main {
// public static void main (String[] args) {
// Scanner t = new Scanner(System.in);
// int a = t.nextInt();
// int b = t.nextInt();
// int sum = 0;
// for(int i=a;a<=b;i++) {
// if (i%2==0) {
// sum -= i;
// System.out.print("-"+i);
// }
// else {
// sum += i;
// if (a==i) {
// System.out.print(i);
// }
// else {
// System.out.print("+"+i);
// }
// }
// if (i==b) {
// break;
// }
// }
// System.out.print("="+sum);
// }
//}
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int x = t.nextInt();
// for (int i=1;i<=x;i++) {
// for (int j=1; j<=x; j++) {
// System.out.print("*");
// }
// System.out.println();
// }
// }
//}
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int x = t.nextInt();
// for (int i=1;i<=x;i++) {
// for(int j=1;j<=x;j++) {
// if(i==1||i==x) {
// System.out.print("*");
// }
// else if(j==1||j==x) {
// System.out.print("*");
// }
// else {
// System.out.print(" ");
// }
// }
// System.out.println();
// }
// }
//}
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int a = t.nextInt();
// for (int i=1;i<=a;i++) {
// for (int j=1;j<=a;j++) {
// if(i==1||i==a) {
// System.out.print("*");
// }
// else if(j==1||j==a) {
// System.out.print("*");
// }
// else if (j+i==a+1) {
// System.out.print("*");
// }
// else if(i-j==0) {
// System.out.print("*");
// }
// else {
// System.out.print(" ");
// }
// }
// System.out.println();
// }
// }
//}
import java.util.*;
public class Main {
public static void main(String[] args) {
Scanner t = new Scanner(System.in);
int l = t.nextInt();
for(int i=1;i<=l;i++) {
for(int j=1;j<=l;j++) {
if(i==1||i==l) {
System.out.print("*");
}
else if(j==1||j==l) {
System.out.print("*");
}
else if(j+i==l+1) {
System.out.print("*");
}
else if(i-j==0) {
System.out.print("*");
}
else if(j==l/2+1) {
System.out.print("*");
}
else if((l+1)/2==i) {
System.out.print("*");
}
else {
System.out.print(" ");
}
}
System.out.println();
}
}
}
0
0
2
27woojaej
2024년 7월 10일
In 소스 코드 제출
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int a = t.nextInt();
// int b = t.nextInt();
// int c = t.nextInt();
// int d = a*b;
// for(int i=c;;i=b*i++) {
// int e = 0;
// int f = i*d;
// int g = f+e;
// System.out.println(g);
// break;
// }
// }
//}
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int a = t.nextInt();
// for(int i=1;i<=9;i++) {
// for (int j = 1; j <= i * a; j++) {
// System.out.print("*");
// }
// System.out.println();
// }
// }
//}
/*
import java.util.*;
public class Main {
public static void main(String[] args) {
Scanner t = new Scanner(System.in);
int a = t.nextInt();
int d = t.nextInt();
int n = t.nextInt();
for(int i=0; i<n-1; i++) {
a += d;
}
System.out.println(a);
}
}
*/
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// long a = t.nextLong();
// int r = t.nextInt();
// int n = t.nextInt();
// for(int i=0; i<n-1; i++) {
// a *= r;
// }
// System.out.println(a);
// }
//}
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// long a = t.nextLong();
// int m = t.nextInt();
// int d = t.nextInt();
// int n = t.nextInt();
// for(int i=0; i<n-1; i++) {
// a = a*m+d;
// }
// System.out.println(a);
// }
//}
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// double capital = t.nextInt();
// double x = capital;
// int day = t.nextInt();
// for (int i=1; i<=day; i++) {
// int percentage = t.nextInt();
// double profit = (capital)*(percentage/100.0);
// capital += profit;
// }
// System.out.printf("%.0f\n",capital-x);
//
// if (capital-x>0) {
// System.out.println("good");
// }
// else if (capital-x==0) {
// System.out.println("same");
// }
// else {
// System.out.println("bad");
// }
// }
//}
/**
*
* 5명 점수가 제일 높은 사람 찾기
* max= 0점
* 1번학생 3점 -> max와 3점 비교 -> max= 3
* 2번학생 1점 -> max와(3점과) 1점 비교
* 3번학생 29점 -> max와(3점과) 29점 비교 -> max= 29
* 4번학생 31점 -> max와(29점과) 31점 비교 -> max= 31
* 5번학생 21점 -> max와(31점과) 21점 비교
*
* >>> 두둥 5명중 가장 높은 점수는 31점입니다!!!
*/
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int a = t.nextInt();
// int max = 0;
// for (int i=1; i<=a;i++) {
// // b : i번째 학생의 점수
// int b = t.nextInt();
//
// // max보다 b가 크다면?
// if (max<b) {
// max=b;
// }
// }
// System.out.println(max);
// }
//}
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int n = t.nextInt();
// int max = 0;
// int min = 1000;
// for (int i=1;i<=n;i++) {
// int x = t.nextInt();
// if (min>x ) {
// min=x;
// }
// else if (max<x) {
// max=x;
// }
// }
// System.out.println(max-min);
// }
//}
0
0
1
27woojaej
2024년 7월 03일
In 소스 코드 제출
//20240703
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int a = t.nextInt();
// int b = 2012 - a+1;
//
// System.out.print(b%100);
//
//
// if (b < 2000) {
// System.out.println(" 1");
// }
// else if (b >= 2000) {
// System.out.println(" 3");
// }
// }
//}
//a b c d
//0 0 1 1
//0 1 1 0
//1 1 0 0
//1 0 0 1
//0 1 0 1
/*
import java.util.*;
public class Main {
public static void main(String[] args) {
Scanner t = new Scanner(System.in);
int a = t.nextInt();
int b = t.nextInt();
int c = t.nextInt();
int d = t.nextInt();
if (a+b+c+d==1) {
System.out.println("도");
}
else if (a+b+c+d==2) {
System.out.println("개");
}
else if (a+b+c+d==3) {
System.out.println("걸");
}
else if (a+b+c+d==4) {
System.out.println("윷");
}
else if (a+b+c+d==0) {
System.out.println("모");
}
}
}
조건문
1. if-elseif-else
2. switch-case
반복문
1. for 50%
2. while 49%
3. do-while 1%
*/
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
//// int a = t.nextInt();
//// for(;;) {
//// System.out.println("안녕!");
//// }
//
//
//// if(a==1) {
//// System.out.println("안녕!");
//// }
//// int i=1;
//// while(i<=5) {
//// System.out.println(i+"번째 놀이기구 탑승을 환영합니다!");
//// i=i+1;
//// }
////
//// System.out.println(" 당신은 6번째 타기를 시도하셨습니다. 집에가세요. The End...");
////
//// int i;
//// // 6번 반복해야지, 8번 반복해야지,
//// for( i=1 ; i<=5 ; i=i+1 ) {
//// System.out.println(i+"번째 놀이기구 탑승을 환영합니다!");
//// }
////
//// // 반복하다가 어떤 조건에 마주했을때 나가세요
//// while(가방이 가득차지 않았다면) {
////
//// }
//// i=i+1; -> i++;
//// i=i-1; -> i--;
//
// for(int i= 5; i>=1;i--) {
// System.out.println(i+" ");
// }
//
//
//
//
//
// }
//}
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// for(int i =1; i<=100; i++) {
// System.out.println(i+"");
// }
// }
//}
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int a = t.nextInt();
// for(int i =1; i<=a; i++) {
// System.out.print(i+" ");
// }
// }
//}
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int a = t.nextInt();
// int b = t.nextInt();
//
// if(a<=b) {
// for(int i=a;i<=b;i++) {
// System.out.println(i+"");
// }
// }
// else {
// for(int i=b;i<=a;i++) {
// System.out.println(i+"");
// }
// }
// }
//}
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// double a = t.nextDouble();
// double b = t.nextDouble();
// for(double i=a;i<=b;i=i+0.01) {
// System.out.printf("%.2f ",i);
// }
// }
//}
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int a = t.nextInt();
// int b = t.nextInt();
// for(int i=a;i<=b;i=i+1) {
// if(i%2!=0) {
// System.out.print(i+" ");
// }
// }
// }
//}
//sum
//cnt
//max
//min
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int a = t.nextInt();
// for(int i=1;i<=9;i++) {
// System.out.println(a+"*"+i+"="+a*i);
// }
//
// }
//}
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int n = t.nextInt();
// int sum = 0;
// for(int i=1;i<=n;i++) {
// sum = i+sum;
// }
// System.out.println(sum);
// }
//}
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int a = t.nextInt();
// int sum = 0;
// for(int i=1;i<=a;i++) {
// if(i%2==0) {
// sum = i+sum;
// }
// }
// System.out.println(sum);
// }
// }
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int a = t.nextInt();
// int b = t.nextInt();
// int sum = 0;
// for(int i=a;i<=b;i++) {
// if(i%3==0) {
// sum = sum+i;
// }
// }
// System.out.println(sum);
// }
// }
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int n = t.nextInt();
// int pro = 1;
// for(int i= n; i>=1;i--) {
// pro = i*pro;
// }
// System.out.println(pro);
// }
//} n을 k번 곱한 것을 말한다.
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int n = t.nextInt();
// int k = t.nextInt();
// int squ = 1;
// for (int i=1; i<=k;i++) {
// squ = squ*n;
// }
// System.out.println(squ);
//
// }
//}
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int a= t.nextInt();
// for (int i=1; i<=a;i++) {
// if (a%i==0) {
// System.out.print(i+" ");
// }
// }
// }
//}
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int a = t.nextInt();
// int cnt = 0;
// for (int i=1; i<=a;i++) {
// if (a%i==0) {
// cnt = cnt+1;
// }
// }
// if (cnt==1||cnt>2) {
// System.out.println("not prime");
// }
// else if (cnt==2) {
// System.out.println("prime");
// }
// }
//}
import java.util.*;
public class Main {
public static void main(String[] args) {
Scanner t = new Scanner(System.in);
int a = t.nextInt();
int sum = 0;
for (int i=1; i<=a;i++) {
//1. b에 정수 입력받기
int b = t.nextInt();
//2. sum에 b 더하기
sum = b+sum;
}
//3.sum 출력하기
System.out.println(sum);
}
0
0
4
27woojaej
2024년 6월 28일
In 소스 코드 제출
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int a = t.nextInt();
// int b = t.nextInt();
// int c = t.nextInt();
// if (a<=170||b<=170||c<=170) {
// System.out.println("CRASH");
// }
//
// else {
// System.out.println("PASS");
// }
// }
//}
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int a = t.nextInt();
// int b = t.nextInt();
// if ((90-a)%5!=0) {
// System.out.println(1+b+(90-a)/5);
// }
// if ((90-a)%5==0) {
// System.out.println(b+(90-a)/5);
// }
// }
//}
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// double a = t.nextDouble();
// double b = t.nextDouble();
// double c = a-100;
// double d = (a-150)/2+50;
// double e = (a-100)*0.9;
// if (a<150) {
// if ((b-c)*100/c<=10) {
// System.out.println("정상");
// }
// else if (10<((b-c)*100)/c&&((b-c)*100)/c<=20) {
// System.out.println("과체중");
// }
// else if (20<(b-c)*100/c) {
// System.out.println("비만");
// }
// }
// if (150<=a&&a<160) {
// if (((b-d)*100)/d<=10) {
// System.out.println("정상");
// }
// else if (10<((b-d)*100)/d&&((b-d)*100)/d<=20) {
// System.out.println("과체중");
// }
// else if (20<(b-d)*100/d) {
// System.out.println("비만");
// }
// }
// if (160<=a) {
// if (((b-e)*100)/e<=10) {
// System.out.println("정상");
// }
// else if (10<((b-e)*100)/e&&((b-e)*100)<=20) {
// System.out.println("과체중");
// }
// else if (20<((b-e)*100)/e) {
// System.out.println("비만");
// }
// }
// }
// }
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int a = t.nextInt();
// int b = t.nextInt();
// int c = t.nextInt();
// if (a>170&&b>170&&c>170) {
// System.out.println("PASS");
// }
// if (a<=170) {
// System.out.println("CRASH"+" "+a);
// }
// else if (b<=170) {
// System.out.println("CRASH"+" "+b);
// }
// else if (c<=170) {
// System.out.println("CRASH"+" "+c);
// }
// }
//}
//import java.util.*;
//
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
//
// double x = t.nextDouble();
// double y = t.nextDouble();
//
// double stand = 0;
// double fat = 0;
// if( x < 150 ) {
// stand = (x - 100);
// }
// else if( x < 160 ) {
// stand = (x - 150) / 2 + 50;
// }
// else {
// stand = (x - 100) * 0.9;
// }
//
// fat = (y - stand) * 100 / stand;
//
// if(fat <= 10) {
//
// }
// else if(fat <= 20) {
//
// }
// else {
//
// }
// }
//}
//
//
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int a = t.nextInt();
// int b = t.nextInt();
// int c = t.nextInt();
// int t = 0;
// if (a>b) {
// t = a;
// a = b;
// b = t;
// }
// }
//}
//
//
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int order1 = t.nextInt();
// int order2 = t.nextInt();
//
// int x = 0;
//
// if(order1 == 1) {
// x += 400;
// }
// else if(order1 == 2) {
// x += 340;
// }
// else if(order1==3) {
// x += 170;
// }
// else if(order1==4) {
// x += 100;
// }
// else if(order1==5) {
// x += 70;
// }
//
// if(order2 == 1) {
// x += 400;
// }
// else if(order2 == 2) {
// x += 340;
// }
// else if(order2==3) {
// x += 170;
// }
// else if(order2==4) {
// x += 100;
// }
// else if(order2==5) {
// x += 70;
// }
//
// if(x > 500) {
// System.out.println("angry");
// }
// else {
// System.out.println("no angry");
// }
// }
//}
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int a = t.nextInt();
// int b = t.nextInt();
// int c = t.nextInt();
// if (a>=b&&b>=c||a>c&&c>b) {
// if (a<b+c) {
// System.out.println("yes");
// }
// else {
// System.out.println("no");
// }
// }
// else if (b>=c&&c>=a||b>a&&a>c) {
// if (b<c+a) {
// System.out.println("yes");
// }
// else {
// System.out.println("no");
// }
// }
// else if (c>=a&&a>=b||c>b&&c>a) {
// if (c<a+b) {
// System.out.println("yes");
// }
// else {
// System.out.println("no");
// }
// }
// }
//}
//import java.util.*;
//
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
//
// int a = t.nextInt();
// int b = t.nextInt();
// int c = t.nextInt();
//
// if(a > b) {
// int x = a;
// a = b;
// b = x;
// }
// if(b > c) {
// int x = b;
// b = c;
// c = x;
// }
//
// if(c < a+b) {
// System.out.println("YES");
// }
// else {
// System.out.println("NOOO");
// }
// }
//}
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int a = t.nextInt();
// if (a>=90) {
// System.out.println("A"); }
// else if (a>=80) {
// System.out.println("B"); }
// else if (a>=70) {
// System.out.println("C"); }
// else if (a>=60) {
// System.out.println("D"); }
// else if (a<60) {
// System.out.println("F"); }
//
// }
// }
//
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int a = t.nextInt();
// int b = t.nextInt();
// int c = t.nextInt();
// if (a>=b&&b>=c) {
// System.out.println(b); }
// else if (a>=c&&c>=b) {
// System.out.println(c); }
// else if (b>=a&&a>=c) {
// System.out.println(a); }
// else if (b>=c&&c>=a) {
// System.out.println(c); }
// else if (c>=a&&a>=b) {
// System.out.println(a); }
// else if (c>=b&&b>=a) {
// System.out.println(b); }
// }
//}
//
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int a = t.nextInt();
// int b = t.nextInt();
// int c = t.nextInt();
// if ((90-a)%5!=0) {
// if ((90-a)/5+b+1>c) {
// System.out.println("win"); }
// else if ((90-a)/5+b+1==c) {
// System.out.println("same"); }
// else if ((90-a)/5+b+1<c) {
// System.out.println("lose"); }
// }
// if ((90-a)%5==0) {
// if ((90-a)/5+b>c) {
// System.out.println("win"); }
// else if ((90-a)/5+b==c) {
// System.out.println("same"); }
// else if ((90-a)/5+b<c) {
// System.out.println("lose"); }
// }
// }
//}
//
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int a = t.nextInt();
// int b = t.nextInt();
// int c = t.nextInt();
// if (b-c>a) {
// System.out.println("advertise"); }
// else if (b-c==a) {
// System.out.println("does not matter"); }
// else if (b-c<a) {
// System.out.println("do not advertise"); }
// }
// }
0
0
2
27woojaej
2024년 6월 26일
In 소스 코드 제출
//import java.util.*;
//
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int a = t.nextInt();
// int b = t.nextInt();
// int c = t.nextInt();
// if (c > b && b > a) {
// System.out.println(a+" "+b+" "+c);
// }
// if (a==b&&b==c) {
// System.out.println(a+" "+b+" "+c);
// }
// if (a>b&&b>=c) {
// System.out.println(c+" "+b+" "+a);
// }
// if (a > c && c > b) {
// System.out.println(b+" "+c+" "+a);
// }
// if (a>=b&& b>c) {
// System.out.println(c+" "+b+" "+a);
// }
// if (b >= a && a > c) {
// System.out.println(c+" "+a+" "+b);
// }
// if (b >= c && c > a) {
// System.out.println(a+" "+c+" "+b);
// }
// if (c >= a && a > b) {
// System.out.println(b+" "+a+" "+c);
// }
// if (c >= b && b > a) {
// System.out.println(a+" "+b+" "+c);
// }
// if (a>b&& b>=c) {
// System.out.println(c+" "+b+" "+a);
// }
// if (b > a && a>=c) {
// System.out.println(c+" "+a+" "+b);
// }
// if (b > c && c >= a) {
// System.out.println(a+" "+c+" "+b);
// }
// if (c > a && a >= b) {
// System.out.println(b+" "+a+" "+c);
// }
// if (c > b && b >= a) {
// System.out.println(a+" "+b+" "+c);
// }
// }
//}
//import java.util.*;
//
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int a = t.nextInt();
// int b = t.nextInt();
// int c = t.nextInt();
// if (a>=b&&a>=c) {
// System.out.println(c+" "+b+" "+a);
// }
// else {
// System.out.println(b+" "+c+" "+a);
// }
// }
//}
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int a = t.nextInt();
// if ((a%10*10+a/10)*2%100<=50) {
// System.out.println((a%10*10+a/10)*2%100);
// System.out.println("GOOD");
// }
// else {
// System.out.println((a%10*10+a/10)*2%100);
// System.out.println("OH MY GOD");
// }
// }
//}
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int a = t.nextInt();
// int b = t.nextInt();
// if (b>=30) {
// System.out.println(a+" "+(b-30));
// }
// else {
// if(a!=0) {
// System.out.println(a-1+" "+(b+30));
// }
// else {
// System.out.println((a+23)+" "+(b+30));
// }
// }
// }
//}
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int a = t.nextInt();
// int b = t.nextInt();
// if (b==1||b==3||b==5||b==7||b==8||b==10||b==12) {
// System.out.println("31");
// }
// else if (b==4||b==6||b==9||b==11) {
// System.out.println("30");
// }
// if (a%400==0&&b==2) {
// System.out.println("29");
// }
// else if (a%4==0&&a%100!=0&&b==2) {
// System.out.println("29");
// }
// else if (b==2) {
// System.out.println("28");
// }
//
// }
// }
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int a = t.nextInt();
// if (a%10==1&&a!=11) {
// System.out.println(a+"st");
// }
// else if (a%10==2&&a!=12) {
// System.out.println(a+"nd");
// }
// else if (a%10==3&&a!=13) {
// System.out.println(a+"rd");
// }
// else {
// System.out.println(a+"th");
// }
//
// }
//
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int a = t.nextInt();
// int b = t.nextInt();
// int c = t.nextInt();
// if (a+b>c) {
// if (a==b&& b==c) {
// System.out.println("정삼각형");
// }
// else if (a==b||b==c||c==a) {
// System.out.println("이등변삼각형");
// }
// else if (c*c==a*a+b*b) {
// System.out.println("직각삼각형");
// }
// }
// else {
// System.out.println("삼각형아님");
// }
// }
//}
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int a = t.nextInt();
// int b = t.nextInt();
// if (b==1||b==2) {
// System.out.println(2012-(1900+(a/10000))+1);
// }
// if (b==3||b==4) {
// System.out.println(2012-(2000+(a/10000))+1);
// }
// }
//}
0
0
3
27woojaej
2024년 6월 21일
In 소스 코드 제출
// 6월 21일은 여기부터
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int h = t.nextInt();
// int b = t.nextInt();
// int c = t.nextInt();
// int s = t.nextInt();
// System.out.printf("%.1f",(double) h*b*c*s/8/1024/1024);
// System.out.print(" "+ "MB");
// }
//}
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int a = t.nextInt();
// int b = t.nextInt();
// System.out.printf("%.1f",(float)a*b/2);
// }
//}
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int a = t.nextInt();
// int b = t.nextInt();
// if (a>b) {
// System.out.println(">");
// }
// else if (a<b) {
// System.out.println("<");
// }
// else if (a==b) {
// System.out.println("=");
// }
// }
//}
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int a = t.nextInt();
// if (a%7==0) {
// System.out.println("multiple");
// }
// else {
// System.out.println("not multiple");
// }
// }
//}
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// double a = t.nextDouble();
// if (50<=a&&a<=60) {
// System.out.println("win");
// }
// else {
// System.out.println("lose");
// }
// }
//}
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int a = t.nextInt();
// int b = t.nextInt();
// if (a%2==0) {
// System.out.print("짝수");
// }
// else if (a%2!=0) {
// System.out.print("홀수");
// }
// System.out.print("+");
// if (b%2==0) {
// System.out.print("짝수");
// }
// else if (b%2!=0) {
// System.out.print("홀수");
// }
// System.out.print("=");
// if ((a+b)%2==0) {
// System.out.print("짝수");
// }
// else {
// System.out.print("홀수");
// }
// }
//}
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int YY = t.nextInt();
// int MM = t.nextInt();
// int DD = t.nextInt();
// if ((YY-MM+DD)%10==0) {
// System.out.println("대박");
// }
// else {
// System.out.println("그럭저럭");
// }
// }
//}
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int YY = t.nextInt();
// int MM = t.nextInt();
// int DD = t.nextInt();
// if ((YY+MM+DD)%2==0) {
// System.out.println("대박");
// }
// else {
// System.out.println("그럭저럭");
// }
// }
//}
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int a = t.nextInt();
// int b = t.nextInt();
// int c = t.nextInt();
// if (a<=170||b<=170||c<=170) {
// System.out.println("CRASH");
// }
//
// else {
// System.out.println("PASS");
// }
// }
//}
import java.util.*;
public class Main {
public static void main(String[] args) {
Scanner t = new Scanner(System.in);
int a = t.nextInt();
int b = t.nextInt();
if ((90-a)%5!=0) {
System.out.println(1+b+(90-a)/5);
}
if ((90-a)%5==0) {
System.out.println(b+(90-a)/5);
}
}
}
0
0
2
27woojaej
2024년 6월 19일
In 소스 코드 제출
//import java.util.*;
//
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
//
// String a = t.nextLine();
//
// System.out.println(a);
// }
// }
//산술연산자 (계산)
//+ - * / %
//
//a+b
//a-b
//a*b
//a/b 정수/정수 -> 정수몫
//ex) 10/3 -> 3
//실수/정수
//정수/실수 -> 실수값
//실수/실수
//ex)10.0/3 -> 3.333333
//a%b a를 b로 나눈 나머지
//ex) 10%3 -> 1
//int : 약 -21억 ~ +21억 (-2147483648 ~ 2147483647)
//long : int 보다는 큰 범위, 무한대는 아님
//overflow (오버플로 )
//
//int + int -> int
//long + int
//int + long -> long
//long + long
//
//casting 강제형변환
/*
import java.util.*;
public class Main{
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int a = sc.nextInt();
int b = sc.nextInt();
// System.out.println((long)a+b);
System.out.println(a/b); // 정수/정수 -> 정수 몫
System.out.println((float)a/b); //실수/정수 -> 실수
System.out.println(a%b);
}
}
1042-1047
*/
//
//import java.util.*;
//
//public class Main {
// public static void main(String[] args ) {
// Scanner t = new Scanner(System.in);
//
// int a = t.nextInt();
// int b = t.nextInt();
//
// System.out.println(a/b);
//
// }
//}
//
//
//
//import java.util.*;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
// int a = t.nextInt();
// int b = t.nextInt();
// System.out.println(a%b);
// }
//}
/*
import java.util.*;
public class Main {
public static void main(String[] args) {
Scanner t = new Scanner(System.in);
int a = t.nextInt();
int b = t.nextInt();
int c = t.nextInt();
System.out.println(a+b+c);
System.out.printf("%.1f",(float)(a+b+c)/3);
}
}
*/
/*
import java.util.*;
public class Main {
public static void main(String[] args) {
Scanner t = new Scanner(System.in);
int a = t.nextInt();
System.out.println((long)a*2);
}
}
*******************************
비교 > < >= <= == !=
1. 결과는 true or false로 나온다
2. >= <= != =을 항상 오른쪽에
3. == vs =
a=1; (대입) a에 1을 대입해.
a==1 (비교) a와 1이 같나요?
우재=2;
우재==2
**********************************
논리연산자
논리값 (true or false)
1. not !
a = true;
!a -> false
2. and && -> 양쪽 모두 true인가요?
( ) && ( )
a b a && b
false false false
false true false
true false false
true true true
ex)
int a=10;
int b=5;
System.out.println(a==10 && b>5);
3. or || -> 둘 중 하나라도 true인가요?
a b a || b
false false false
false true true
true false true
true true true
************************************
import java.util.*;
public class Main {
public static void main(String[] args) {
int a=1,b=7; // a에 1을 대입해 (명령)
if (a==b) {
System.out.println();
}
else if(a>b) {
}
else {
}
}
}
*/
/*
import java.util.*;
public class Main {
public static void main(String[] args) {
Scanner t = new Scanner(System.in);
int a = t.nextInt();
int b = t.nextInt();
if (a>b) {
System.out.println("1");
}
else if (a<=b) {
System.out.println("0");
}
}
}
*/
/*
import java.util.*;
public class Main {
public static void main(String[] args) {
Scanner t = new Scanner(System.in);
int a = t.nextInt();
int b = t.nextInt();
if (a==b) {
System.out.println("1"); }
else {
System.out.println("0");
}
}
}
*/
/*
import java.util.*;
public class Main {
public static void main(String[] args) {
Scanner t = new Scanner(System.in);
int a = t.nextInt();
int b = t.nextInt();
if (b>=a) {
System.out.println("1"); }
else {
System.out.println("0");
}
}
}
*/
/*
import java.util.*;
public class Main {
public static void main(String[] args) {
Scanner t = new Scanner(System.in);
int a = t.nextInt();
int b = t.nextInt();
if (a==b) {
System.out.println("0"); }
else {
System.out.println("1");
}
}
}
*/
/*
import java.util.*;
public class Main {
public static void main(String[] args) {
Scanner t = new Scanner(System.in)70
;
int a = t.nextInt();
if (a==1) {
System.out.println("0"); }
else if (a==0) {
System.out.println("1"); }
}
}
*/
/*
import java.util.*;
public class Main {
public static void main(String[] args) {
Scanner t = new Scanner(System.in);
int a = t.nextInt();
int b = t.nextInt();
if (a==1 && b==1) {
System.out.println("1"); }
else {
System.out.println("0"); }
}
}
*/
/*
import java.util.*;
public class Main {
public static void main(String[] args) {
Scanner t = new Scanner(System.in);
int a = t.nextInt();
int b = t.nextInt();
if ( a==1||b==1) {
System.out.println("1"); }
else {
System.out.println("0");
}
}
}
*/
/*
import java.util.*;
public class Main {
public static void main(String[] args) {
Scanner t = new Scanner(System.in);
int a = t.nextInt();
int b = t.nextInt();
System.out.println(a + "+" + b + "=" +(a+b));
System.out.println(a + "-" + b + "=" +(a-b));
System.out.println(a + "*" + b + "=" +(a*b));
System.out.println(a + "/" + b + "=" +(a/b));
}
}
*/
/*
import java.util.*;
public class Main {
public static void main(String[] args) {
Scanner t = new Scanner(System.in);
double a = t.nextDouble();
double b = t.nextDouble();
System.out.printf("%.2f",a*b);
}
}
*/
/*
import java.util.*;
public class Main {
public static void main(String[] args) {
Scanner t = new Scanner(System.in);
int a = t.nextInt();
System.out.println(a/60 + " " +(a%60));
}
}
산술연산자 + - * / % , overflow, casting
비교연산자 > < >= <= == != true or false,
논리연산자 ! && ||
삼항연산자 (조건연산자)
조건식 ? 조건식이 true일때의 값 : 조건식이 false일때의 값
조건식 (결과가 true or false로 나오는 식)
int c = 50>70 ? 100 : 200 ;
// a가 10보다 크면 hello, 아니면 world 를 출력
System.out.println( a>10 ? "hello" : "world" );
if(a>10) {
System.out.println("hello");
}
else {
System.out.println("world");
}
import java.util.*;
public class Main {
public static void main(String[] args) {
Scanner t = new Scanner(System.in);
int a = t.nextInt();
int b = t.nextInt();
// 둘 중 큰 수 ? 둘 중 작은 수 ? 주로 사용
int box = a>b ? a : b ;
System.out.println(box);
int box2 = a<b ? a:b ;
// Scanner t = new Scanner(System.in);
// double a = t.nextDouble();
// System.out.printf("%.3f",1.8*(a)+32);
}
}
*/
/*
import java.util.*;
public class Main {
public static void main(String[] args) {
Scanner x = new Scanner(System.in);
int a = x.nextInt();
int b = x.nextInt();
System.out.println(a>b? a:b);
}
}
*/
/*
import java.util.*;
public class Main {
public static void main(String[] args) {
Scanner t = new Scanner(System.in);
int a = t.nextInt();
int b = t.nextInt();
int c = t.nextInt();
int box = a<b? a:b;
System.out.println(box<c? box:c);
}
}
*/
/*
import java.util.*;
public class Main {
public static void main(String[] args) {
Scanner t = new Scanner(System.in);
int a = t.nextInt();
int b = t.nextInt();
System.out.println(a%b);
}
}
*/
/*
import java.util.*;
public class Main {
public static void main(String[] args) {
Scanner t = new Scanner(System.in);
int a = t.nextInt();
System.out.println(-a);
}
}
*/
0
0
6
27woojaej
2024년 6월 14일
In 소스 코드 제출
//
//public class Main {
// public static void main(String[] args) {
// System.out.println("Hello");
// System.out.println("World");
// }
//}
//public class Main {
// public static void main(String[] args) {
// System.out.println("\"C:\\Download\\hello.cpp\"");
// }
//}
//import java.util.*;
//
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
//
// char a = t.next().charAt(0);
//
// System.out.println(a);
// }
//}
//
/*
정수형
int
long
실수형
float
double
문자형
char
문장형
string
*/
// import java.util.*;
//
// public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
//
// double a = t.nextDouble();
//
// System.out.printf("%.6f", a);
// }
// }
// import java.util.*;
//
// public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
//
// int a = t.nextInt();
//
// int b = t.nextInt();
//
// System.out.println(a);
// System.out.println(b);
// }
// }
//
//
// import java.util.*;
//
// public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
//
// char a = t.next().charAt(0);
// char b = t.next().charAt(0);
//
// System.out.println(b);
// System.out.println(a);
// }
// }
//
//
//
// import java.util.*;
//
// public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
//
// double a = t.nextDouble();
//
// System.out.printf("%.2f", a);
//
//
// }
// }
//
//
//
import java.util.*;
public class Main {
public static void main(String[] args) {
Scanner t = new Scanner(System.in);
int a = t.nextInt();
System.out.println(a + " " + a + " " + a);
// System.out.printf("%d %d %d", a, a, a);
}
}
0
0
8
27woojaej
더보기
bottom of page