'''
recursion is when you put the same function inside the same function, calling it out to do the same work.
works like an overcomplicated for문 but you can also do 재귀 multiple times!
just put the function name and ( whatever you want to use recursion on ) and the value inside brackets will be put inside the function!
'''
#
#
#
# EXAMPLE ONE = 1->5, printing backwards (since the command is -1. a little harder)
# btw i made a mistake in writing explanations. printed would be between 1~5 not 0~5 bc of the if n==0, return command.
#
#
def rec(n): # 함수 introduction (name can be anything)
if n == 0: # 조건 가장먼저 두는것이 좋다! (BEFORE 재귀, setting that will end the recursion)
return
rec(n-1) # here is the RECURSION = 재귀, like for문 but "holds the position" for use after return
print(n) # a command, this will ONLY HAPPEN AFTER THE RECURSION IS COMPLETED / BROKEN
rec(5)
'''
this is how the upper command will work.
because the function rec's 재귀 is BEFORE the print command,
the computer will just hold place of the 5->0 numbers.
after hitting the if n==0 / return command,
it will return backwards up to 0->5,
while following the print(n) command.
rec(5):
rec(4):
rec(3):
rec(2):
rec(1):
rec(0):
if ~~~:
return
end
print(1)
end
print(2)
end
print(3)
end
print(4)
end
print(5)
'''
#
#
# EXAMPLE TWO: 5-> 1, printing forwards (since the command is n-1)
#
#
'''
opposite version of this (printing 5->0):
'''
def rec2(n):
if n == 0:
return
print(n)
rec(n-1)
rec2(5)
'''
since this defined function has print command before the recursion command,
printing happens before the recursion and so the "return command makes the function print backwards" is gone.
sot it would print 5->0 while saving the recursion positions but there are no commands after recursion so nothing happens
and when it gets to 0 it hits return and just ends each saved recursion positions without doing anything
rec(5):
print(5)
rec(4):
print(4)
rec(3):
print(3)
rec(2):
print(2)
rec(1):
print(1)
rec(0):
if ~~~:
return
end
end
end
end
end
'''
top of page
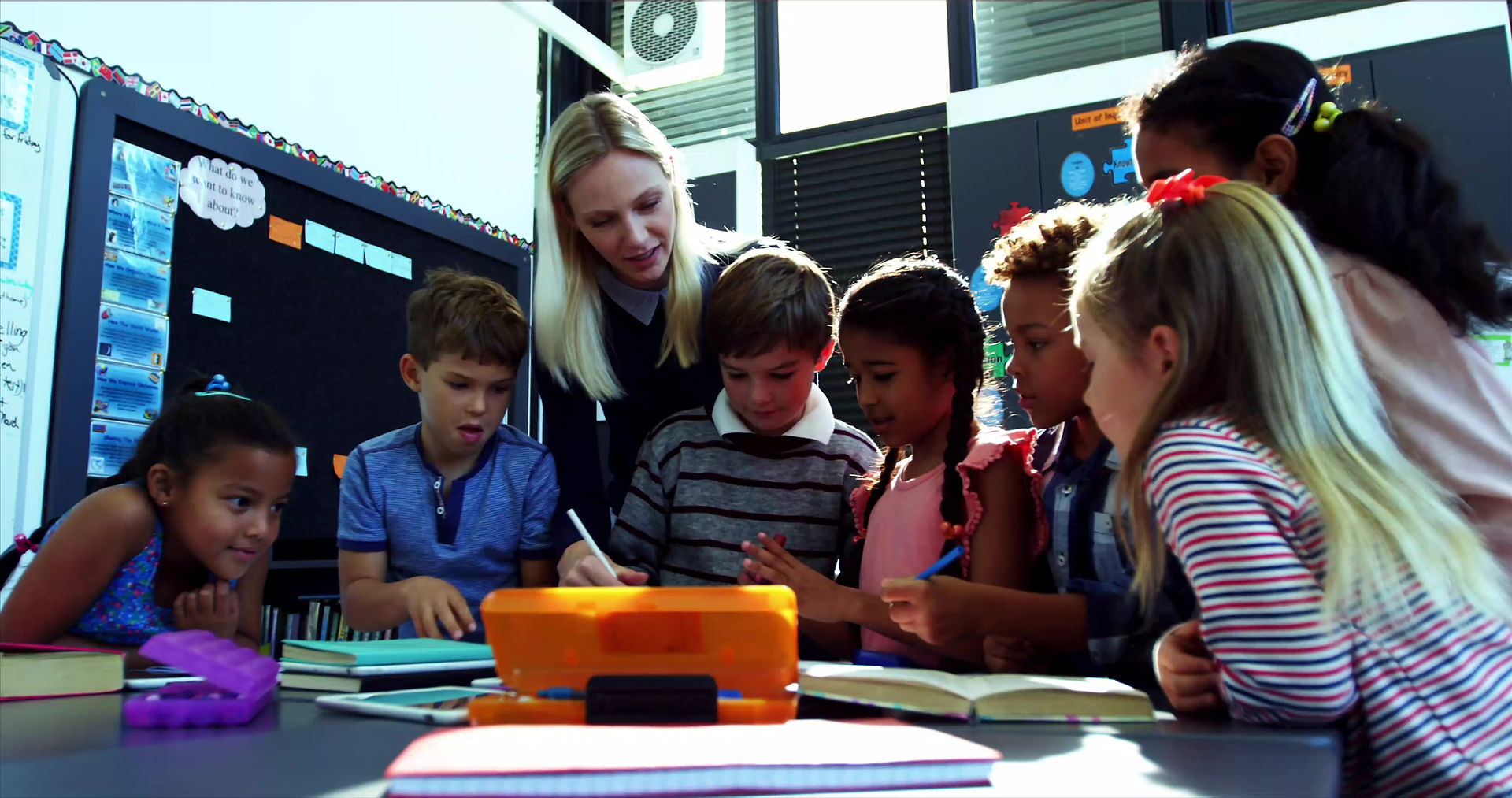
실제 작동 상태를 확인하려면 라이브 사이트로 이동하세요.
recursion 20240721
recursion 20240721
댓글 0개
좋아요
댓글(0)
bottom of page