import javax.swing.*;
import java.awt.*;
/*
* Container (= Content Pane): JFrame, JPanel
* JFrame and JPanel Classes have INHERITED the Container Class.
* JFrame is like a desk, JPanel is like a blank paper on the desk.
*/
public class Main extends JFrame {
public Main() {
setTitle("Hazel you're so cool");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// closes the program when frame is exited
// another way to exit any Java program:
// System.exit(0);
Container contentPane = getContentPane();
// 컨텐트팬 (Container) 을 알아낸다
contentPane.setBackground(Color.pink);
contentPane.setLayout(new FlowLayout());
// Layout manager just helped me arrange my components into a free-flow layout! From left to right.
// 1. Always remember to add "new" layout.
// 2. You can change the layout of both a container (content pane) OR a panel.
contentPane.setLayout(new FlowLayout(FlowLayout.LEFT, 30, 40));
// Can change 정렬방법 by:
// FlowLayout(alignment, horizontal pixel gap, vertical pixel gap);
// 3 alignments: FlowLayout.LEFT, FlowLayout.RIGHT, FlowLayout.CENTER (default)
contentPane.add(new JButton("OK"));
contentPane.add(new JButton("Cancel"));
contentPane.add(new JButton("Ignore"));
JButton omg = new JButton("OMG!!");
omg.setForeground(Color.blue);
contentPane.add(omg);
JButton yippee = new JButton("Yippee~");
contentPane.add(yippee);
yippee.setForeground(Color.green);
// buttonName.setForeground(Color.colorName); <- changes text colour of the button!!
setSize(300, 200);
setVisible(true);
}
public static void main(String[] args) {
new Main();
}
}
top of page
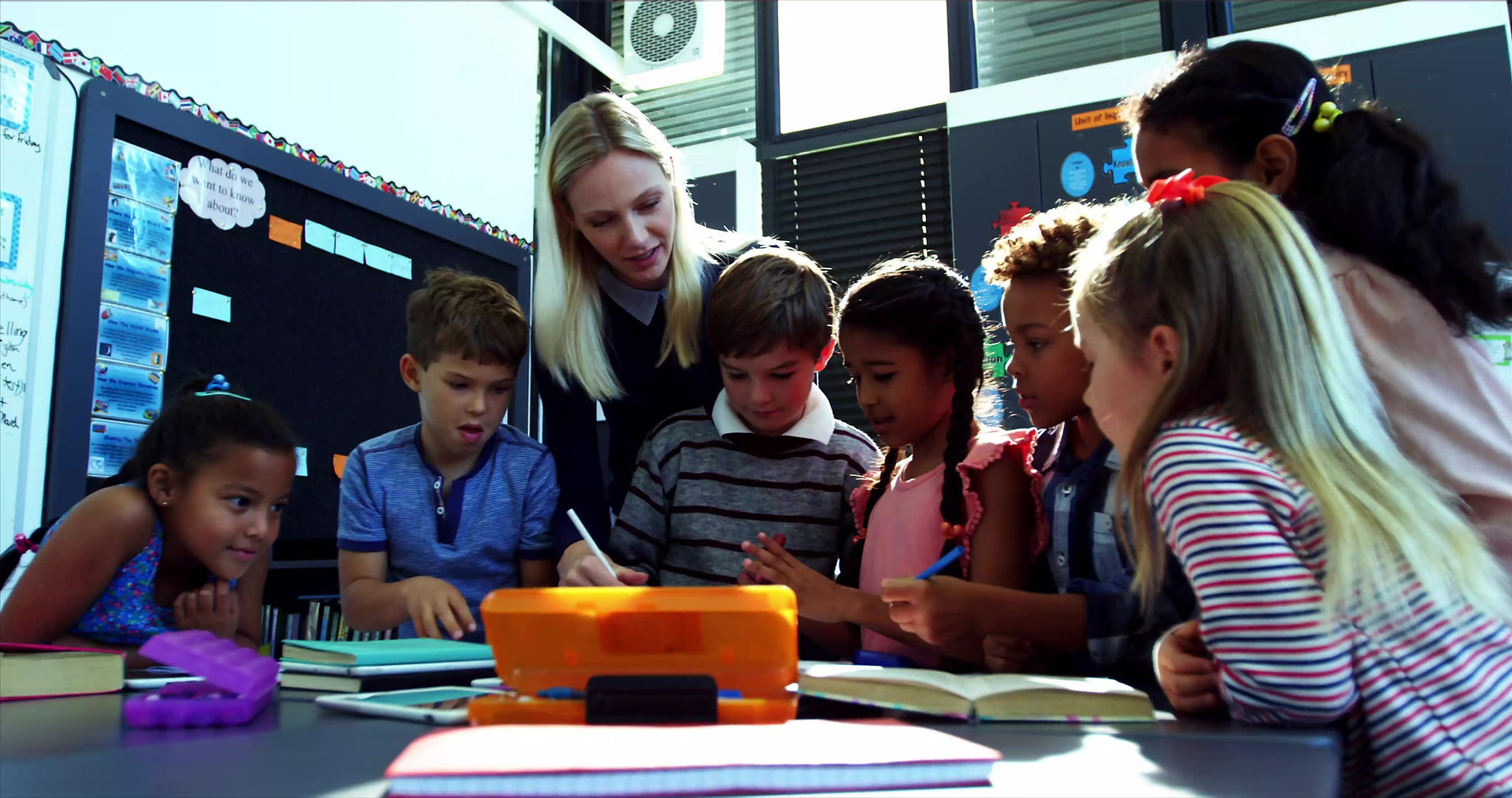
기능을 테스트하려면 라이브 사이트로 이동하세요.
GUI 1: simple button display
GUI 1: simple button display
댓글 2개
좋아요
댓글(2)
bottom of page
When you want to freely layout your display, you can write:
contentPane.setLayout(null); // for containers (= content panes) JPanel p = new JPanel(); p.setLayout(null); // for panels
When you set your layout to null, you have to set the sizes & locations by yourself.
JButton btn = new JButton("Hello"); btn.setSize(100,40); btn.setLocation(50,50); p.add(btn); btn.setBounds(50,50,100,40); // same effect - location AND THEN size