class Item
{
private int ID;
public Item (int id)
{
// constructor
ID = id;
}
public int getID()
{
return ID;
}
public void addToCollection (ItemCollection c)
{
// c here is NOT related to the code segment A, JUST a parameter name that happens to overlap
c.addItem(this);
/*
* calls the addItem method by using its class(ItemCollection)'s object, c
* "this" = inserts the Item object (i and j in code segment A) into addItem
* = self-insert Item object into anther class ItemCollection's method: addItem
*/
}
}
class ItemCollection
{
private int last_ID;
// doesn't have any constructors = default constructor
// the object c in code segment A also uses default constructor
// meaning that last_ID is equal to null (0) in the following steps of addItem method
public void addItem (Item i)
// takes Item i as a parameter, NOT related to the code segment A, JUST an overlapping parameter name
{
if (i.getID() == last_ID)
// uses the getID method in class Item using Item object i to return ID
// compares i's ID to last_ID (at first empty because default constructor)
// so, last_ID would be 0 in ItemCollection object c in code segment A
{
System.out.print("ID " + i.getID() + " rejected; ");
}
else
// if the ID of class Item's object i is NOT equal to the last compared ID, accept
{
last_ID = i.getID();
System.out.print("ID " + i.getID() + " accepted; ");
}
}
}
//////code segment A (written in a separate class other than Item or ItemCollection
// prints "ID 23 accepted; ID 32 accepted; ID 32 rejected; ID 23 accepted;"
class Main{
public static void main(String[] args) {
Item i = new Item(23);
Item j = new Item(32);
ItemCollection c = new ItemCollection();
i.addToCollection(c);
// AFTER it finishes every step in Item and ItemCollection back and forth, move on to next line
j.addToCollection(c);
j.addToCollection(c);
i.addToCollection(c);
}
}
// additional info from quiz:
/// static can be updated in different objects's methods and keep its updated value
//// static methods can access/update static variables but not instance variables
///// do not make a parameter name overlap with a pre-existing instance variable
////// don't declare the dataType of an instance variable except in the first initialization step
////// because it will be recognized as a local variable not instance variable
/////// printing an entire object will look for a .toString method (can be separate or attached)
/////// usually it will be written as a separate method, returning String
/////// if .toString isn't specified, will print some jibberish
//////// a method w return type other than "void" MUST have a return value for every possible outcome
///////// getValue should have same return dataType as the value
top of page
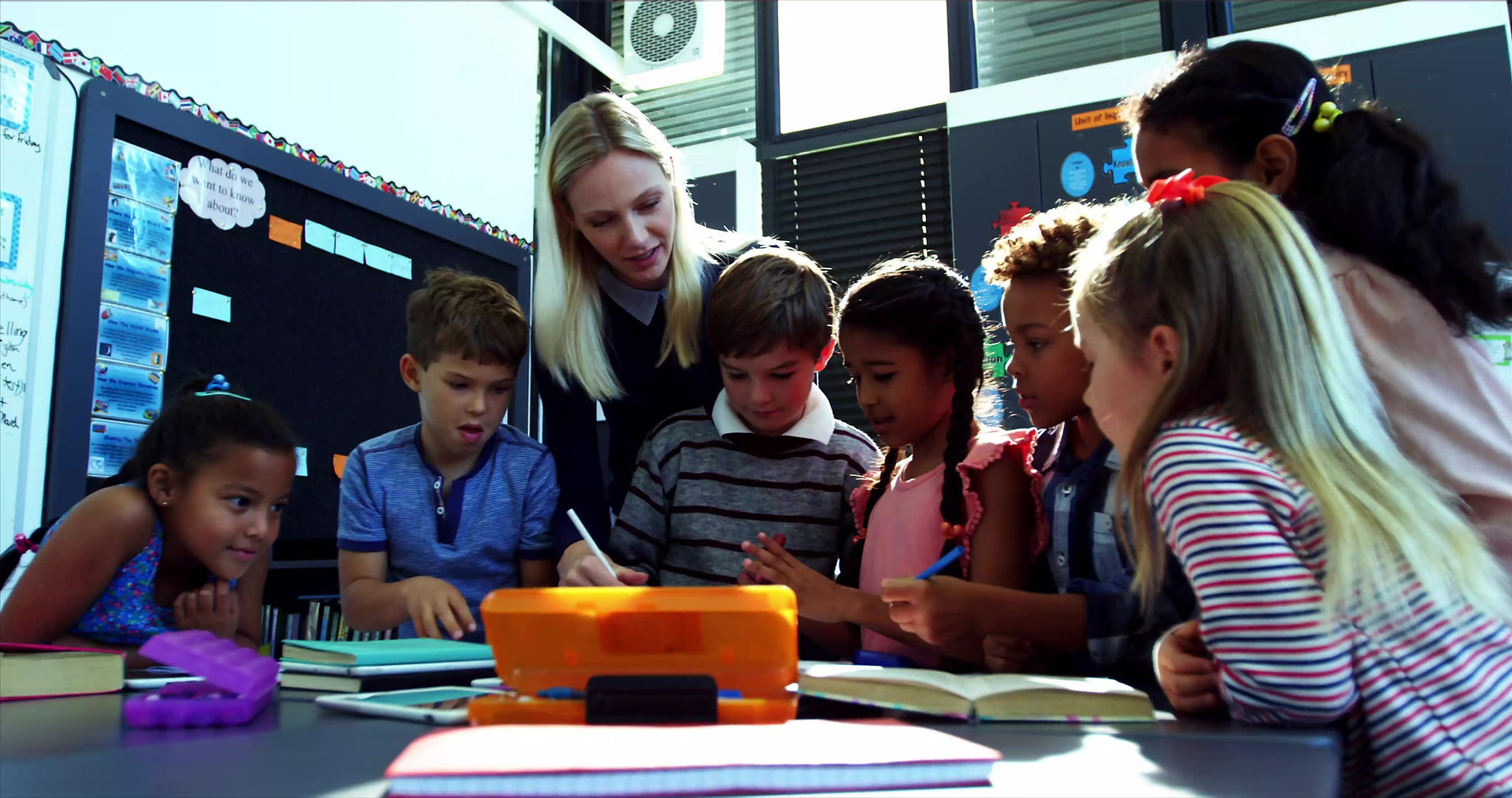
실제 작동 상태를 확인하려면 라이브 사이트로 이동하세요.
수정: 11월 24일
CB this Keyword Quiz Q1 and GOOD CH5 tips
CB this Keyword Quiz Q1 and GOOD CH5 tips
댓글 0개
좋아요
댓글(0)
bottom of page