import sys
sys.setrecursionlimit(10000)
box_list = []
total_box = 0
village_cnt, max_box = map(int,input().split())
box_num = int(input())
for i in range(box_num):
vil_num, send_num, rec_num = map(int,input().split())
box_list.append([vil_num, send_num, rec_num])
print(box_list)
box_list.sort()
print(box_list)
trunk = []
now_loc = 0
in_box = 0
del_list = []
for i in range(box_num):
for j in range(len(trunk)):
if trunk[j][0] == now_loc:
in_box -= trunk[j][1]
del_list.append(j)
for j in range(len(del_list)):
del(trunk[del_list[j]])
if box_list[i][0] != now_loc:
now_loc += 1
if max_box > in_box + box_list[i][2]:
in_box += box_list[i][2]
total_box += box_list[i][2]
trunk.append([box_list[i][1], box_list[i][2]])
else:
plus_box = box_list[i][2]-(max_box - in_box) #plus box 계산 오류
in_box += plus_box
total_box += plus_box
trunk.append([box_list[i][1], box_list[i][2]])
print(total_box)
top of page
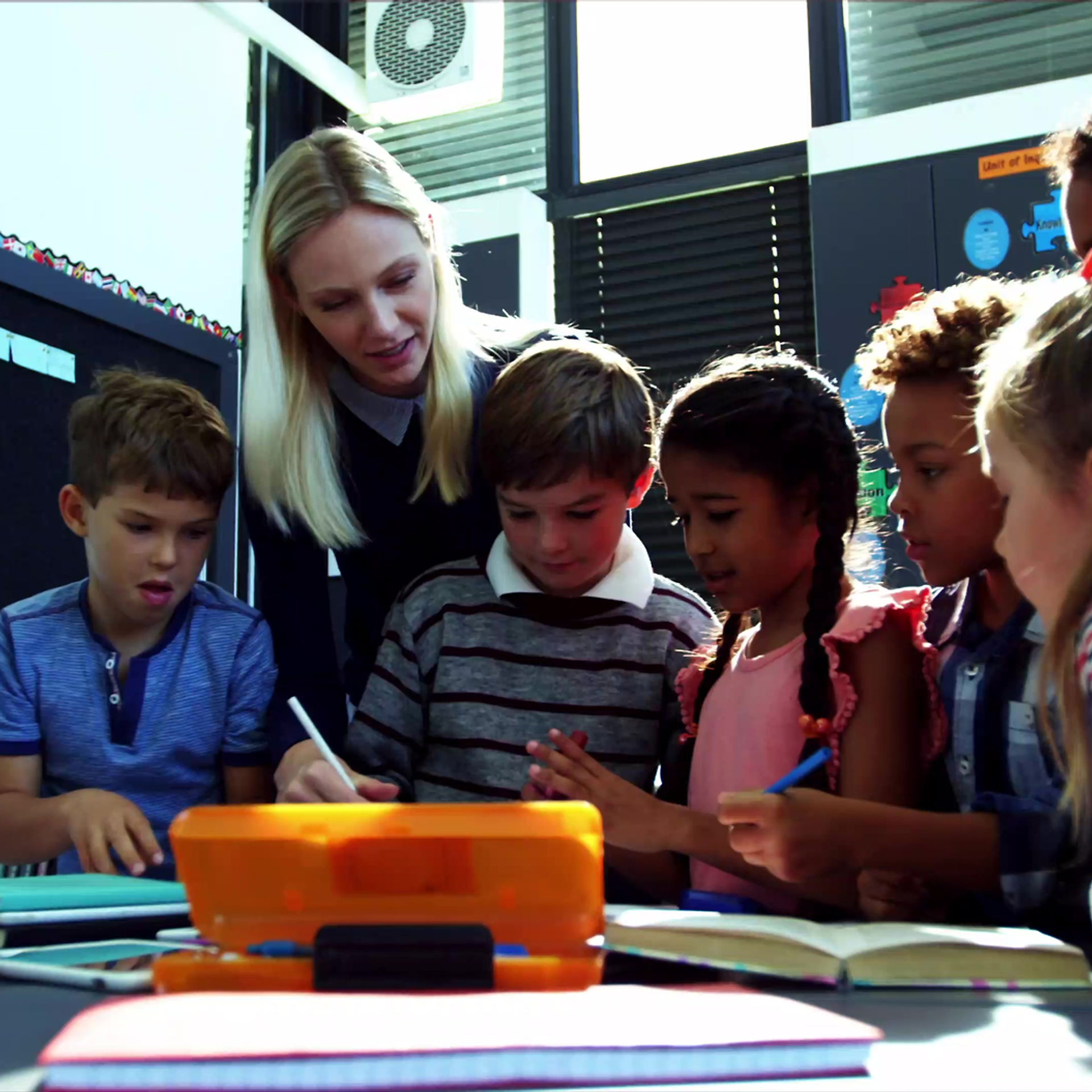
기능을 테스트하려면 라이브 사이트로 이동하세요.
0115
0115
댓글 2개
좋아요
댓글(2)
bottom of page
l, n, k = map(int, input().split()) street = [10000000 for i in range(l)] light = list(map(int, input().split())) min = [1000000 for _ in range(k)] for i in range(len(light)): #가로등 갯수 street[light[i]] = 0 for j in range(1,l): #지역 갯수 if light[i]+j < len(street): if street[light[i] + j] > j: street[light[i]+j] = j if light[i]-j >= 0: if street[light[i] - j] > j: street[light[i]-j] = j print(street) street.sort() for i in range(k): print(street[i])
# import sys # sys.setrecursionlimit(10000) # box_list = [] # total_box = 0 # village_cnt, max_box = map(int,input().split()) # box_capacity = [max_box]*village_cnt # box_num = int(input()) # for i in range(box_num): # vil_num, send_num, rec_num = map(int,input().split()) # box_list.append([vil_num, send_num, rec_num]) # # def efficient_sort(list) : # # new_list = [] # # for i in range(len(list)): # # box_list.sort(key=lambda x : (x[1],x[2])) # print(box_capacity) # for i in range(len(box_list)) : # print(box_capacity) # vil = box_list[i][0] # send = box_list[i][1] # rec_num = box_list[i][2] # mina = min( box_capacity[ vil : send ] ) # minb = min(mina, rec_num) # if minb>0 : # for j in range(vil,send) : # box_capacity[j]-=minb # # box_capacity[vil:send]-=minb # total_box+=minb # print(total_box) # # print(box_list) # # trunk = [] # # now_loc = 0 # # in_box = 0 # # del_list = [] # # for i in range(box_num): # # if box_list[i][0] != now_loc: # # now_loc += 1 # # for j in range(len(trunk)): # # if trunk[j][0] == now_loc: # # in_box -= trunk[j][1] # # del_list.append(j) # # for j in range(len(del_list)): # # del(trunk[del_list[j]]) # # del_list.clear() # # if max_box > in_box + box_list[i][2]: # # in_box += box_list[i][2] # # total_box += box_list[i][2] # # trunk.append([box_list[i][1], box_list[i][2]]) # # else: # # plus_box = (max_box - in_box) #plus box 계산 오류 # # in_box += plus_box # # total_box += plus_box # # trunk.append([box_list[i][1], plus_box]) # # # # print(total_box) n, k = map(int, input().split()) len_list = 0 house_list = [] for i in range(n): x, y = map(int, input().split()) house_list.append([x, y]) def get_s_route(num,max_): global len_list global house_list for i in range(len_list): if house_list[i][0] - house_list[num][0]