# import sys
# from PyQt5.QtWidgets import *
# from PyQt5 import *
# from PyQt5.QtGui import *
# from PyQt5.QtCore import *
# m=0
# m=int(m)
# l=0
# l=int(l)
# o=0
# b=0
# b=int(b)
# def input(n,la1):
# global m
# m=m*10
# m=m+n
# la1.setText(str(m))
# la1.repaint()
# def operator(c,la1):
# global o,m,l,b
# if b==1:
# operate(la1)
# if c=='+':
# o='+'
# if c=='-':
# o='-'
# if c=='*':
# o='*'
# if c=='/':
# o='/'
# if l==0:
# l=m
# m=0
# b=1
# la1.setText(str(m))
# la1.repaint()
# def clear(la1):
# global l,m
# l=0
# m=0
# la1.setText(str(m))
# la1.repaint()
# def operate(la1):
# global l,m,o,b
# a=0
# a=int(a)
# err = 0
# err = int(a)
# if o=='+':
# a=l+m
# if o=='-':
# a=l-m
# if o=='*':
# a=l*m
# if o=='/':
# if m==0:
# err=1
# else:
# a=l/m
# l=a
# m=0
# b=0
# if err==1:
# la1.setText("ERROR 140: ANSWER_UNDEFINED;INPUTS_CLEARED")
# la1.repaint()
# else:
# la1.setText(str(a))
# la1.repaint()
# class MyApp(QWidget):
# global m
# def __init__(self):
# super().__init__()
# def initUI(self):
# lbl1 = QLabel('0', self)
#
# btn1 = QPushButton('&1', self)
# btn1.clicked.connect(lambda: input(1,lbl1))
# btn2 = QPushButton('&2', self)
# btn2.clicked.connect(lambda: input(2, lbl1))
# btn3 = QPushButton('&3', self)
# btn3.clicked.connect(lambda: input(3, lbl1))
# btnp=QPushButton('&+',self)
# btnp.clicked.connect(lambda: operator("+",lbl1))
# btn4 = QPushButton('&4', self)
# btn4.clicked.connect(lambda: input(4, lbl1))
# btn5 = QPushButton('&5', self)
# btn5.clicked.connect(lambda: input(5, lbl1))
# btn6 = QPushButton('&6', self)
# btn6.clicked.connect(lambda: input(6, lbl1))
# btnm = QPushButton('&-', self)
# btnm.clicked.connect(lambda: operator("-", lbl1))
# btn7 = QPushButton('&7', self)
# btn7.clicked.connect(lambda: input(7, lbl1))
# btn8 = QPushButton('&8', self)
# btn8.clicked.connect(lambda: input(8, lbl1))
# btn9 = QPushButton('&9', self)
# btn9.clicked.connect(lambda: input(9, lbl1))
# btnt = QPushButton('&x', self)
# btnt.clicked.connect(lambda: operator("*", lbl1))
# btnc = QPushButton('&C', self)
# btnc.clicked.connect(lambda: clear(lbl1))
# btn0 = QPushButton('&0', self)
# btn0.clicked.connect(lambda: input(0, lbl1))
# btnd = QPushButton('&÷', self)
# btnd.clicked.connect(lambda: operator("/", lbl1))
# btne = QPushButton('&=', self)
# btne.clicked.connect(lambda: operate(lbl1))
# hbox = QHBoxLayout()
# hbox.addStretch(1)
# hbox.addWidget(lbl1)
# hbox.addStretch(0)
# hboxr1=QHBoxLayout()
# hboxr1.addStretch(1)
# hboxr1.addWidget(btn1)
# hboxr1.addWidget(btn2)
# hboxr1.addWidget(btn3)
# hboxr1.addWidget(btnp)
# hboxr1.addStretch(1)
# hboxr2 = QHBoxLayout()
# hboxr2.addStretch(1)
# hboxr2.addWidget(btn4)
# hboxr2.addWidget(btn5)
# hboxr2.addWidget(btn6)
# hboxr2.addWidget(btnm)
# hboxr2.addStretch(1)
#
# hboxr3 = QHBoxLayout()
# hboxr3.addStretch(1)
# hboxr3.addWidget(btn7)
# hboxr3.addWidget(btn8)
# hboxr3.addWidget(btn9)
# hboxr3.addWidget(btnt)
# hboxr3.addStretch(1)
# hboxr4 = QHBoxLayout()
# hboxr4.addStretch(1)
# hboxr4.addWidget(btnc)
# hboxr4.addWidget(btn0)
# hboxr4.addWidget(btnd)
# hboxr4.addWidget(btne)
# hboxr4.addStretch(1)
# vbox = QVBoxLayout()
# vbox.addWidget(lbl1)
# vbox.addStretch(1)
# vbox.addLayout(hboxr1)
# vbox.addLayout(hboxr2)
# vbox.addLayout(hboxr3)
# vbox.addLayout(hboxr4)
# vbox.addStretch(2)
#
# lbl1.setStyleSheet("color: green;"
# "border-style: solid;"
# "border-width: 1px;"
# "border-color: #0000CD;"
# "border-radius: 10px")
# btnp.setStyleSheet("color: black;"
# "background-color: #FF8C00")
# btnm.setStyleSheet("color: black;"
# "background-color: #FF8C00")
# btnt.setStyleSheet("color: black;"
# "background-color: #FF8C00")
# btnd.setStyleSheet("color: black;"
# "background-color: #FF8C00")
# btnc.setStyleSheet("color: black;"
# "background-color: #FFD700")
# btne.setStyleSheet("color: white;"
# "background-color: #4169E1")
# self.setLayout(vbox)
# self.setGeometry(300, 300, 300, 200)
# self.setWindowTitle('Calculator')
# self.show()
# if __name__ == '__main__':
# app = QApplication(sys.argv)
# ex = MyApp()
# ex.initUI()
# sys.exit(app.exec_())
# a=[]
# q=int(0)
# def dfs(x,y,c):
# global a
#
# if x<0 or y<0 or x>=7 or y>=7 or a[x][y]!=c:
# return 0
# a[x][y] = 0
# # print(x,y)
# return dfs(x-1,y,c)+ dfs(x,y-1,c)+dfs(x+1,y,c)+dfs(x,y+1,c)+1
#
# for i in range(7):
# t=list(map(int,input().split()))
# a.append(t)
#
# for i in range(7):
# for j in range(7):
# if a[i][j]!=0 :
# if dfs(i, j, a[i][j])>=3 :
# q+=1
# print(q)
a=[]
n,m=map(int,input().split())
for i in range(n):
t=list(map(int,input().split()))
a.append
top of page
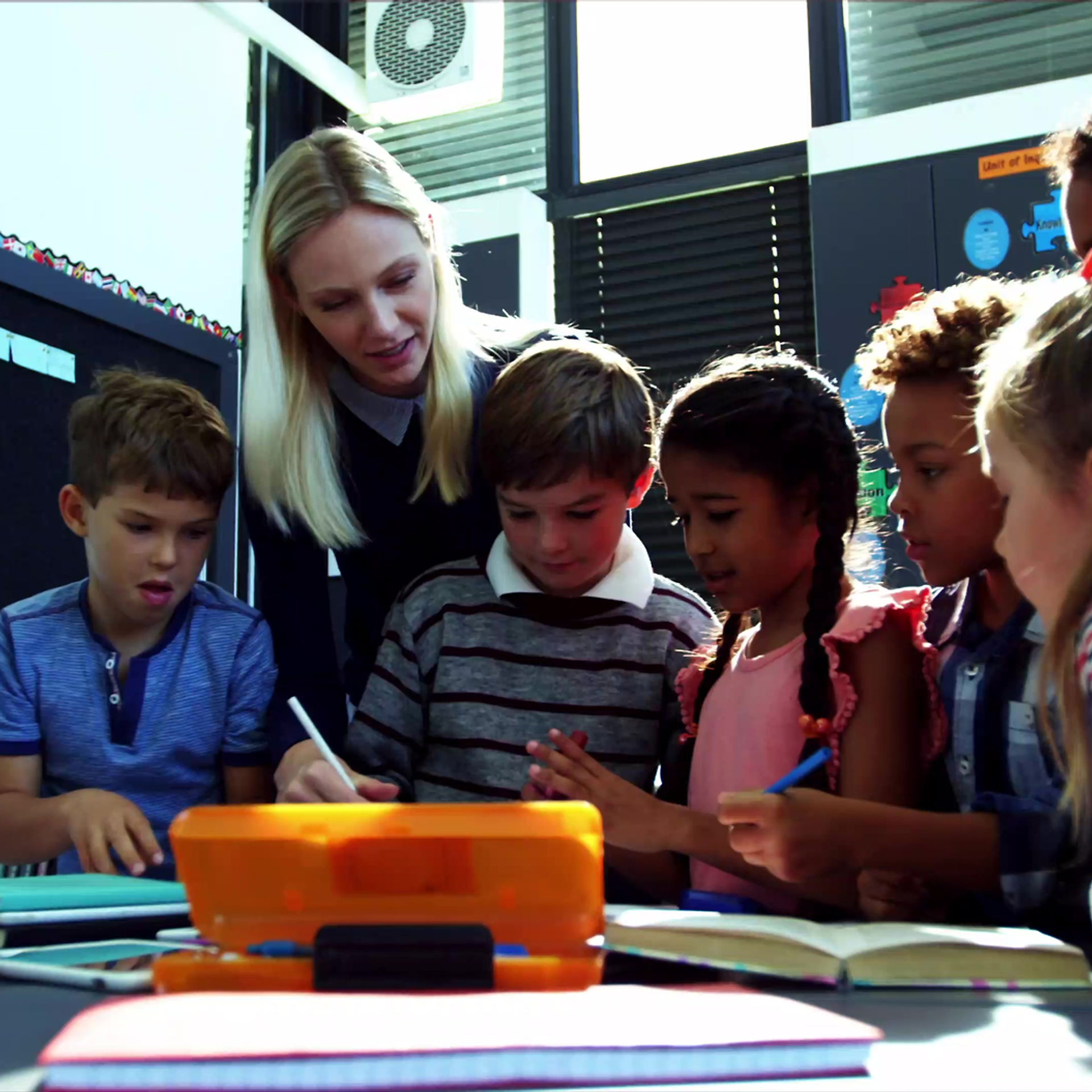
기능을 테스트하려면 라이브 사이트로 이동하세요.
20241217
20241217
댓글 0개
좋아요
댓글(0)
bottom of page