/*
* 프린트할때 항상:
* public class Main {
* public static void main(String[] args) {
* System.out.print("something"); <= printing
* System.out.println("something"); <= printing + press enter
* System.out.print("something\n"); <= printing + press enter
* }
* }
*/
//public class Main {
// public static void main(String[] args) {
// System.out.println("Hello World\n\n\n");
// System.out.println("Apple is GOOOOODOD");
// }
//}
//public class Main {
// public static void main(String[] args) {
// System.out.println("Hello");
// }
//}
//public class Main {
// public static void main(String[] args) {
// System.out.print("Hello ");
// System.out.println("World");
// }
//}
//public class Main {
// public static void main(String[] args) {
// System.out.println("Hello");
// System.out.print("World");
// }
//}
//// SAME THING (new line = put \n together in "")
//public class Main {
// public static void main(String[] args) {
// System.out.print("Hello\nWorld");
// }
//}
//public class Main {
// public static void main(String[] args) {
// System.out.print("\'Hello\'");
// }
//}
//public class Main {
// public static void main(String[] args) {
// System.out.print("\"Hello World\"");
// }
//}
//public class Main {
// public static void main(String[] args) {
// System.out.println("\"!@#$%^&*()\"");
// }
//}
//public class Main {
// public static void main(String[] args) {
// System.out.println("\"C:\\Download\\hello.cpp\"");
// }
//}
/* 변수: 빈상자
*
*
* 정수
* int 32bit, can take on different possiblities
* long 64bit, can take on much more possibilities but heavier
*
* 실수
* float
* double
*
* 문자
* char
* string
*
*
* x (variable input) 쓰고싶다면
* import java.util.Scanner <= at the start
* Scanner t = new Scanner(System.in) <= near the end of the important announcing
*
*
* NEXT QUESTIONS ARE ALL FROM CODEUP "기초2. 입출력문 및 연산자"
*/
//import java.util.Scanner;
//
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner(System.in);
//
//
//// x = 10; if value is already set
//// y = 20; if value is already set
// int x = t.nextInt();
// int y = t.nextInt();
//
// float z = t.nextFloat();
// double p = t.nextDouble();
//
// System.out.println(x + y);
// }
//}
//import java.util.Scanner;
//
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner (System.in);
//
// int x = t.nextInt();
// System.out.println(x);
// }
//}
//import java.util.Scanner;
//
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner (System.in);
//
// char x = t.next().charAt(0);
// System.out.print(x);
// }
//}
/* 문자받을때는 항상 char x = t.next().charAt(0);
* char x <= 변수를 문자형으로 선언
* t.next() 는 그냥 string 프린트할때 쓰는거라서
* charAt(0) 써서 string 말고 맨 앞줄 글자 하나 프린트해야됨
*/
//import java.util.Scanner;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner (System.in);
// float x = t.nextFloat();
// System.out.printf("%.6f", x);
// }
//}
/* 실수 (decimal places):
*
* float x = t.nextFloat();
* System.out.printf("%.6f", x);
*
*
* t.nextFloat() <= just memorize
* USE "printf" WHEN YOU'RE CHANGING INPUT VALUE TO FIT DECIMAL PLACES
* ex. input is 5.3 but you want to print 5.30000
* ("%.6f", x) <= print x in 6 decimal places (default)
*
* if you want to print stuff next to each other with space, just do print(x + " " + y)
*
*
* 산술연산: + - * / %
* unlike python, / does both 몫계산 AND real value 계산
* ex. print(5/2) = 2 (몫계산)
* ex 2. print((float)(5/2)) = 2.5 (real value)
* ex 3. print((double)5/2)) = 2.5 (real value, uses 5 as 5.00)
*
* printing ++x = adding 1 to x and printing (saves as +=1, prints with +=1)
* printing x++ = printing x and adding 1 to it (prints before, later saves as +=1)
*/
//import java.util.Scanner;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner (System.in);
// int x = t.nextInt();
// int y = t.nextInt();
// System.out.print(x + " " + y);
// }
//}
//import java.util.Scanner;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner (System.in);
// char x = t.next().charAt(0);
// char y = t.next().charAt(0);
// System.out.print(y + " " + x);
// }
//}
//import java.util.Scanner;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner (System.in);
// float x = t.nextFloat();
// System.out.printf("%.2f", x);
// }
//}
//import java.util.Scanner;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner (System.in);
// int x = t.nextInt();
// System.out.print(x + " " + x + " " + x);
// }
//}
//import java.util.Scanner;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner (System.in);
// long x = t.nextLong();
// long y = t.nextLong();
// System.out.print(x + y);
// }
//}
//import java.util.Scanner;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner (System.in);
// long x = t.nextLong();
// long y = t.nextLong();
// System.out.print(x+y);
// }
//}
//import java.util.Scanner;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner (System.in);
// long x = t.nextLong();
// System.out.print(-x);
// }
//}
//import java.util.Scanner;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner (System.in);
// char x = t.next().charAt(0);
// System.out.print((char)(x + 1));
// }
//}
//import java.util.Scanner;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner (System.in);
// int x = t.nextInt();
// int y = t.nextInt();
// System.out.print(x/y);
// }
//}
//import java.util.Scanner;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner (System.in);
// int x = t.nextInt();
// int y = t.nextInt();
// System.out.print(x%y);
// }
//}
//import java.util.Scanner;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner (System.in);
// long x = t.nextLong();
// System.out.print(++x);
// }
//}
// TESTING HOW print((double)x/y) WORKS!!
// import java.util.Scanner;
//public class Main {
// public static void main(String[] args) {
// Scanner t = new Scanner (System.in);
// int x = t.nextInt();
// int y = t.nextInt();
// System.out.print((double)x/y);
// }
//}
top of page
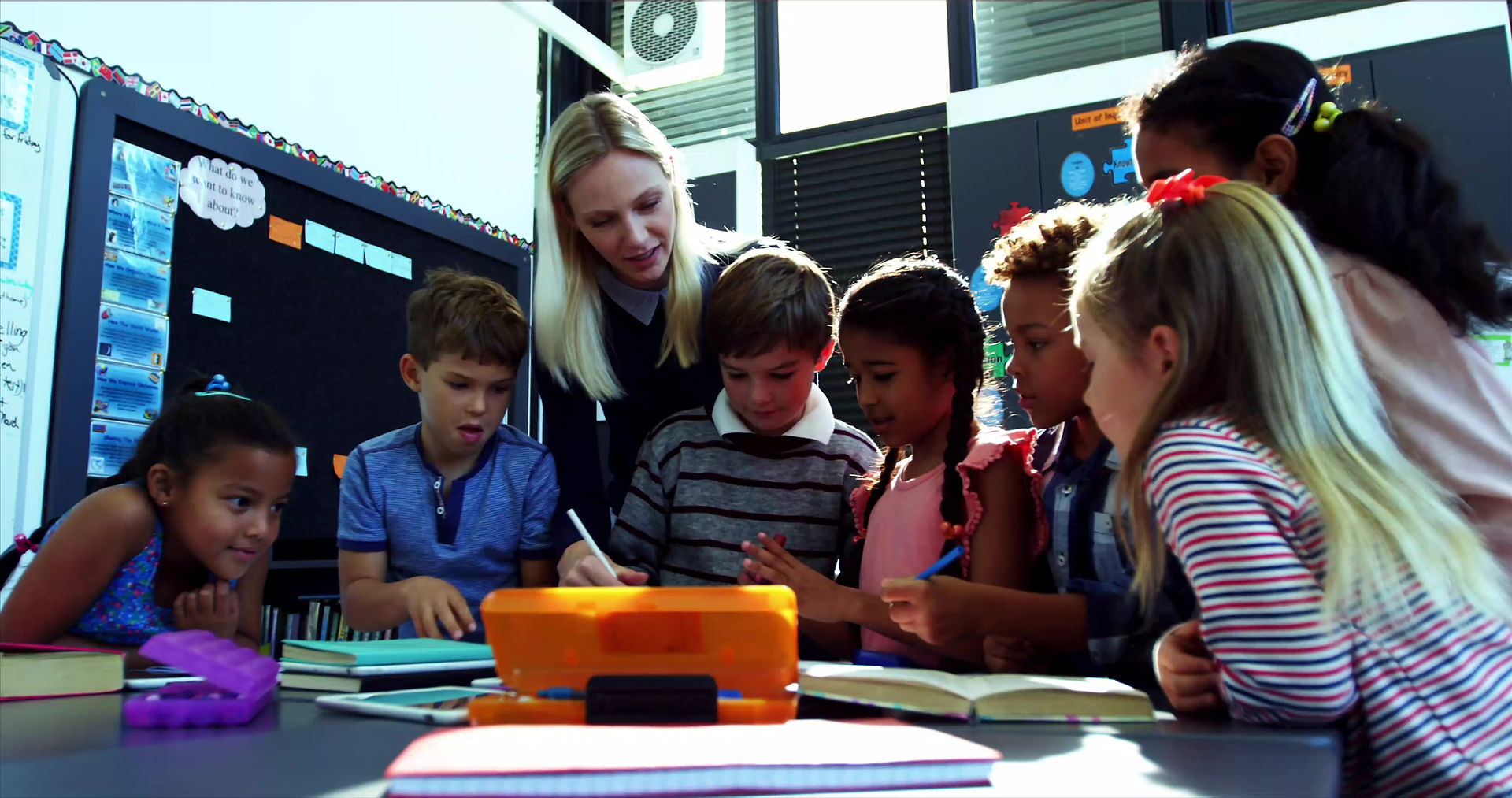
실제 작동 상태를 확인하려면 라이브 사이트로 이동하세요.
20240519 (JAVA START)
20240519 (JAVA START)
댓글 0개
좋아요
댓글(0)
bottom of page