import socket
from _thread import *
import threading
from tkinter import *
from time import sleep
import tkinter.font as tkFont
c_list = []
close = False
server_socket = None
def log_in():
def sendname(socket):
global namebool
global name
name = nametxt.get()
socket.send(name.encode())
namebool = False
def send(socket):
global go_send
while True:
if go_send:
message = (message_input.get(1.0, "end")).rstrip()
socket.send(message.encode())
message_input.delete(1.0, "end")
go_send = False
else:
if go_out:
socket.close()
exit()
sleep(0.1)
def receive(socket):
global space
global name
first = True
global num
global x
global datalist
global datanum
global downbool
while True:
if num < 17:
num += 1
txt = txtParts[num]
datanum += 1
try:
data = socket.recv(1024)
txt['state'] = 'normal'
text = str(data.decode()).split('☈')
if first:
text[0].replace('☈', '').strip()
txt.delete(0, END)
#############################################################################
txt.configure(background='#ececec', disabledbackground='#ececec')
#############################################################################
txt.insert("end", text[0] + text[1])
datalist.append([text[0] + text[1], 'left', 'grey'])
first = False
else:
if str(text[0]) == str(name):
txt.config(justify='right')
txt.delete(0, END)
#############################################################################
txt.configure(background='white', disabledbackground='white')
#############################################################################
txt.insert("end", text[1])
datalist.append([text[1], 'right', 'white'])
elif str(text[0]) == '[System] ':
txt.config(justify='left')
txt.delete(0, END)
#############################################################################
txt.configure(background='#ececec', disabledbackground='#ececec')
#############################################################################
txt.insert("end", text[0] + text[1])
datalist.append([text[0] + text[1], 'left', 'grey'])
else:
text[0].replace('☈', '').strip()
txt.delete(0, END)
txt.config(justify='left')
#############################################################################
txt.configure(background='#ececec', disabledbackground='#ececec')
#############################################################################
txt.insert("end", text[0])
datalist.append([text[0], 'left', 'grey'])
num += 1
if num <= 17:
txtParts[num]['state'] = 'normal'
txtParts[num].delete(0, END)
txtParts[num].config(justify='left')
#############################################################################
txtParts[num].configure(background='white', disabledbackground='white')
#############################################################################
txtParts[num].insert("end", text[1])
datalist.append([text[1], 'left', 'white'])
datanum += 1
txtParts[num]['state'] = 'disabled'
else:
txtParts[17]['state'] = 'normal'
txtParts[17].delete(0, END)
#############################################################################
txtParts[17].configure(background='white', disabledbackground='white')
#############################################################################
datalist.append([text[1], 'left', 'white'])
datanum += 1
downbool = True
go_down()
txt['state'] = 'disabled'
except ConnectionAbortedError as e:
txt['state'] = 'normal'
txt.delete(0, END)
txt.insert("end", '\n[System] 접속을 종료합니다.\n')
txt['state'] = 'disabled'
exit()
else:
txt = txtParts[17]
try:
data = socket.recv(1024)
txt['state'] = 'normal'
text = str(data.decode()).split('☈')
datanum += 1
if first:
text[0].replace('☈', '').strip()
txt.delete(0, END)
#############################################################################
txt.configure(background='#ececec', disabledbackground='#ececec')
#############################################################################
datalist.append([text[0] + text[1], 'left', 'grey'])
downbool = True
go_down()
first = False
else:
if str(text[0]) == str(name):
txt.config(justify='right')
txt.delete(0, END)
#############################################################################
txt.configure(background='white', disabledbackground='white')
#############################################################################
datalist.append([text[1], 'right', 'white'])
downbool = True
go_down()
elif str(text[0]) == '[System] ':
txt.config(justify='left')
txt.delete(0, END)
#############################################################################
txt.configure(background='#ececec', disabledbackground='#ececec')
#############################################################################
txt.insert("end", text[0] + text[1])
datalist.append([text[0] + text[1], 'left', 'grey'])
else:
txt.config(justify='left')
text[0].replace('☈', '').strip()
txt.delete(0, END)
#############################################################################
txt.configure(background='#ececec', disabledbackground='#ececec')
#############################################################################
datalist.append([text[0], 'left', 'grey'])
downbool = True
go_down()
txtParts[17]['state'] = 'normal'
txtParts[17].delete(0, END)
#############################################################################
txtParts[17].configure(background='white', disabledbackground='white')
#############################################################################
datalist.append([text[1], 'left', 'white'])
datanum += 1
downbool = True
go_down()
txtParts[17]['state'] = 'disabled'
txt['state'] = 'disabled'
except ConnectionAbortedError as e:
txt['state'] = 'normal'
txt.delete(0, END)
txt.insert("end", '\n[System] 접속을 종료합니다.\n')
txt['state'] = 'disabled'
exit()
def login():
# 서버의 ip주소 및 포트
HOST = iptxt.get()
PORT = int(porttxt.get())
client_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
client_socket.connect((HOST, PORT))
threading.Thread(target=sendname, args=(client_socket,)).start()
threading.Thread(target=send, args=(client_socket,)).start()
threading.Thread(target=receive, args=(client_socket,)).start()
exit()
def go_up():
global datalist
global updownnum
if updownnum > 0:
updownnum -= 1
for i in range(17, -1, -1):
txtParts[i]['state'] = 'normal'
txtParts[i].delete(0, END)
txtParts[i].insert("end", datalist[i + updownnum][0])
if datalist[i + updownnum][1] == 'right':
txtParts[i].config(justify='right')
else:
txtParts[i].config(justify='left')
#############################################################################
if datalist[i + updownnum][2] == 'grey':
txtParts[i].configure(background='#ececec', disabledbackground='#ececec')
else:
txtParts[i].configure(background='white', disabledbackground='white')
#############################################################################
txtParts[i]['state'] = 'disabled'
def go_down():
global datalist
global updownnum
global datanum
global downbool
print(datanum, updownnum)
if updownnum + 18 <= datanum:
print(':' + str(updownnum))
print(';' + str(datanum) + '\n')
updownnum += 1
for i in range(0, 18):
txtParts[i]['state'] = 'normal'
if datalist[i + updownnum][1] == 'right':
txtParts[i].config(justify='right')
else:
txtParts[i].config(justify='left')
txtParts[i].delete(0, END)
txtParts[i].insert("end", datalist[i + updownnum][0])
#############################################################################
if datalist[i + updownnum][2] == 'grey':
txtParts[i].configure(background='#ececec', disabledbackground='#ececec')
else:
txtParts[i].configure(background='white', disabledbackground='white')
#############################################################################
txtParts[i]['state'] = 'disabled'
# if downbool == True:
# txtParts[17]['state'] = 'normal'
# txtParts[17].config(justify='right')
# txtParts[17].delete(0, END)
# txtParts[17].insert("end", datalist[17 + updownnum])
# txtParts[17]['state'] = 'disabled'
# downbool = False
def set_go_send(event):
global go_send
go_send = True
c_root = Toplevel(root)
c_root.geometry('500x610')
c_root.title('client')
c_root.resizable(False, False)
c_root['bg'] = '#BECDFF'
root.withdraw()
start_new_thread(login, ())
frame = Frame(c_root, width=300)
upbtn = Button(frame, text='up', command=go_up)
upbtn.pack(fill='x')
chat_canvas = Canvas(frame)
chat_canvas.pack()
downbtn = Button(frame, text='down', command=go_down)
downbtn.pack(fill='x')
txtParts = []
txtfont = tkFont.Font(size=10)
for i in range(0, 18):
x = Entry(chat_canvas, width=65, disabledforeground='black')
# x = Entry(chat_canvas, width=65, disabledbackground='#BECDFF', disabledforeground='black', background='#BECDFF')
x.pack()
x.configure(font=txtfont)
x['state'] = 'disabled'
txtParts.append(x)
frame.place(x=10, y=5)
message_frame = Frame(c_root)
message_input = Text(message_frame, width=57, height=7)
message_input.pack(side='left')
send_button = Button(message_frame, text='보내기', command=lambda: set_go_send(None))
send_button.pack(side='right', fill='y')
message_frame.place(x=10, y=500)
# message_input.bind("<Return>", set_go_send)
c_root.mainloop()
go_out, go_send = False, False
root = Tk()
root.resizable(False, False)
root.title('로그인')
root.geometry("300x125-500+200")
namebool = True
space = ''
num = -1
x = True
datalist = []
datanum = -1
downbool = False
updownnum = 0
iplbl = Label(root, text="server IP", width=10)
iplbl.grid(row=0, column=0)
iptxt = Entry(root, width=20)
iptxt.insert(0, '127.0.0.1')
iptxt.grid(row=0, column=1)
portlbl = Label(root, text="port", width=10)
portlbl.grid(row=1, column=0)
porttxt = Entry(root, width=20)
porttxt.insert(0, '9999')
porttxt.grid(row=1, column=1)
namelbl = Label(root, text="이름", width=10)
namelbl.grid(row=2, column=0)
nametxt = Entry(root, width=20)
nametxt.grid(row=2, column=1)
btnlogin = Button(root, text="로그인", command=log_in)
btnlogin.grid(row=3, column=0, columnspan=2, sticky=W + E + N + S)
root.mainloop()
top of page
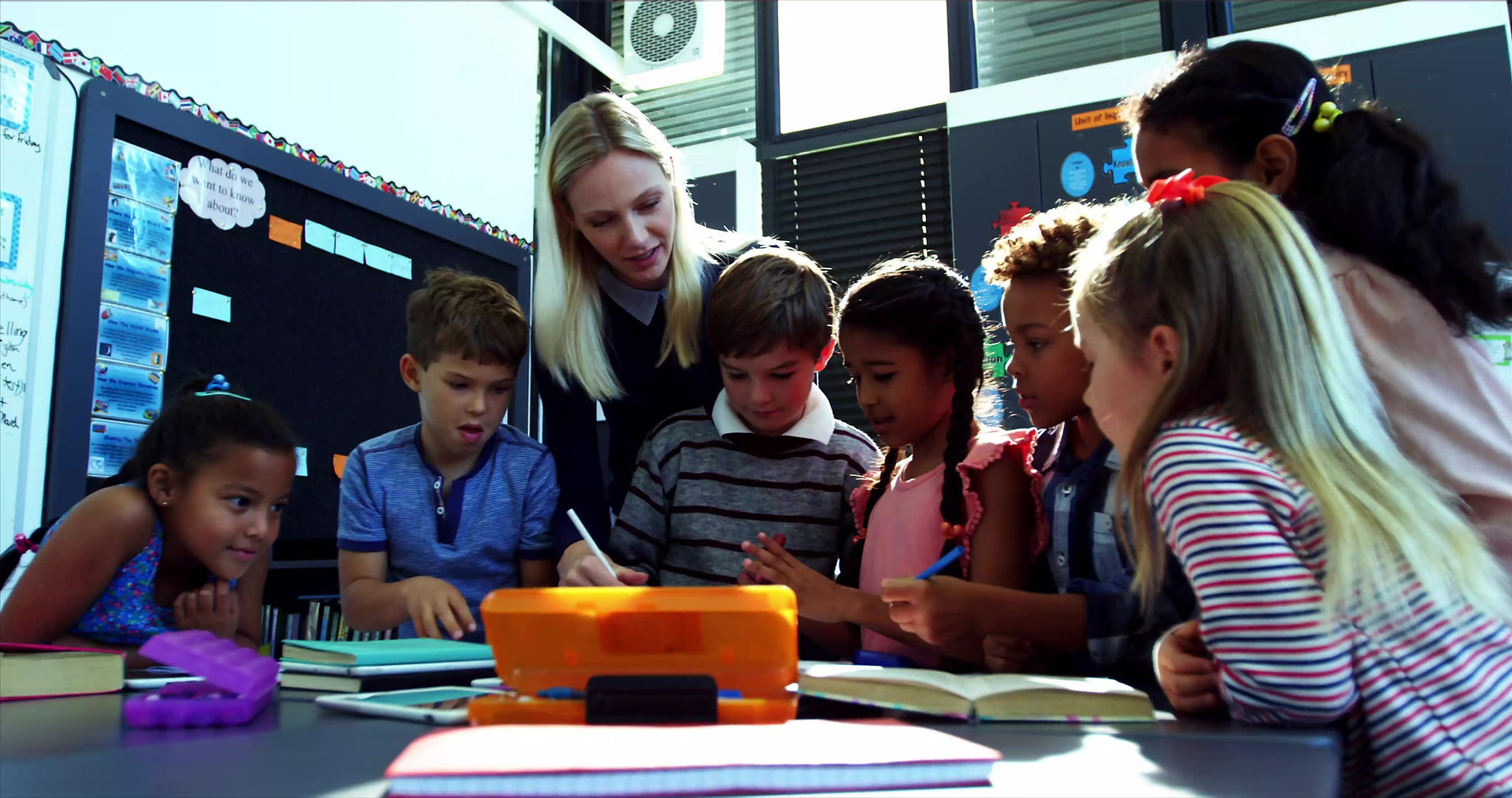
기능을 테스트하려면 라이브 사이트로 이동하세요.
수정: 2022년 10월 11일
2022.10.11.(m)
2022.10.11.(m)
댓글 0개
좋아요
댓글(0)
bottom of page