"""
result = 0
def adder(num):
global result
result += num
return result
print(adder(3))
print(adder(4))
"""
"""
result1 = 0
result2 = 0
def adder1(num):
global result1
result1 += num
return result1
def adder2(num):
global result2
result2 += num
return result2
print(adder1(3))
print(adder1(4))
print(adder2(3))
print(adder2(7))
"""
"""
class Calculator:
def_init_(self):
self.result = 0
def adder(self,num):
self.result += num
return self.result
cal1 = calculator()
cal2 = calculator()
print(cal1.adder(3))
print(cal1.adder(4))
print(cal2.adder(3))
print(cal2.adder(7))
"""
"""
class Service:
secret = "바보"
def sum(self, a, b):
result = a + b
print("%s + %s = %s입니다." %(a, b, result))
def minus(self, a, b):
result = a - b
print("%s - %s = %s입니다." %(a, b, result))
def multiple(self, a, b):
result = a * b
print("%s * %s = %s입니다." %(a, b, result))
def divide(self, a, b):
result = a / b
print("%s / %s = %s입니다." %(a, b, result))
pey = Service()
print(pey.secret)
pey.sum(2, 1)
pey.minus(2, 1)
pey.multiple(2,1)
pey.divide(2,1)
"""
"""
class Service:
secret = "바보"
def setname(self,name):
self.name = name
def sum(self, a, b):
result = a + b
print("%s님 %s + %s = %s입니다." %(self.name, a, b, result))
pey = Service()
pey.setname("Sharon")
pey.sum(2, 1)
"""
"""
class Service:
secret = "바보"
def __init__(self, name):
self.name = name
def sum(self, a, b):
result = a + b
print("%s님 %s + %s = %s입니다." %(self.name, a, b, result))
pey = Service("Sharon")
pey.sum(2, 1)
"""
"""
class Service:
def sum(self, a, b):
result = a + b
print("%s + %s = %s입니다." %(a, b, result))
def minus(self, a, b):
result = a - b
print("%s - %s = %s입니다." %(a, b, result))
def multiple(self, a, b):
result = a * b
print("%s * %s = %s입니다." %(a, b, result))
def divide(self, a, b):
result = a / b
print("%s / %s = %s입니다." %(a, b, result))
pey = Service()
input = input().split()
a = int(input[0])
b = int(input[2])
op = input[1]
if op == '+':
pey.sum(a, b)
elif op =='-':
pey.minus(a, b)
elif op =='*':
pey.multiple(2,1)
elif op =='/':
pey.divide(2,1)
"""
"""
data = []
for i in range(0, 9):
inside = input().split()
array = []
for j in range (0, len(inside)):
array.append(int(inside[j]))
data.append(array)
max = 0
x = 0
y = 0
for i in range(0, 9):
for j in range(0, 9):
if data[i][j] > max:
max = data[i][j]
x = i
y = j
print(max)
print(x+1, y+1)
"""
top of page
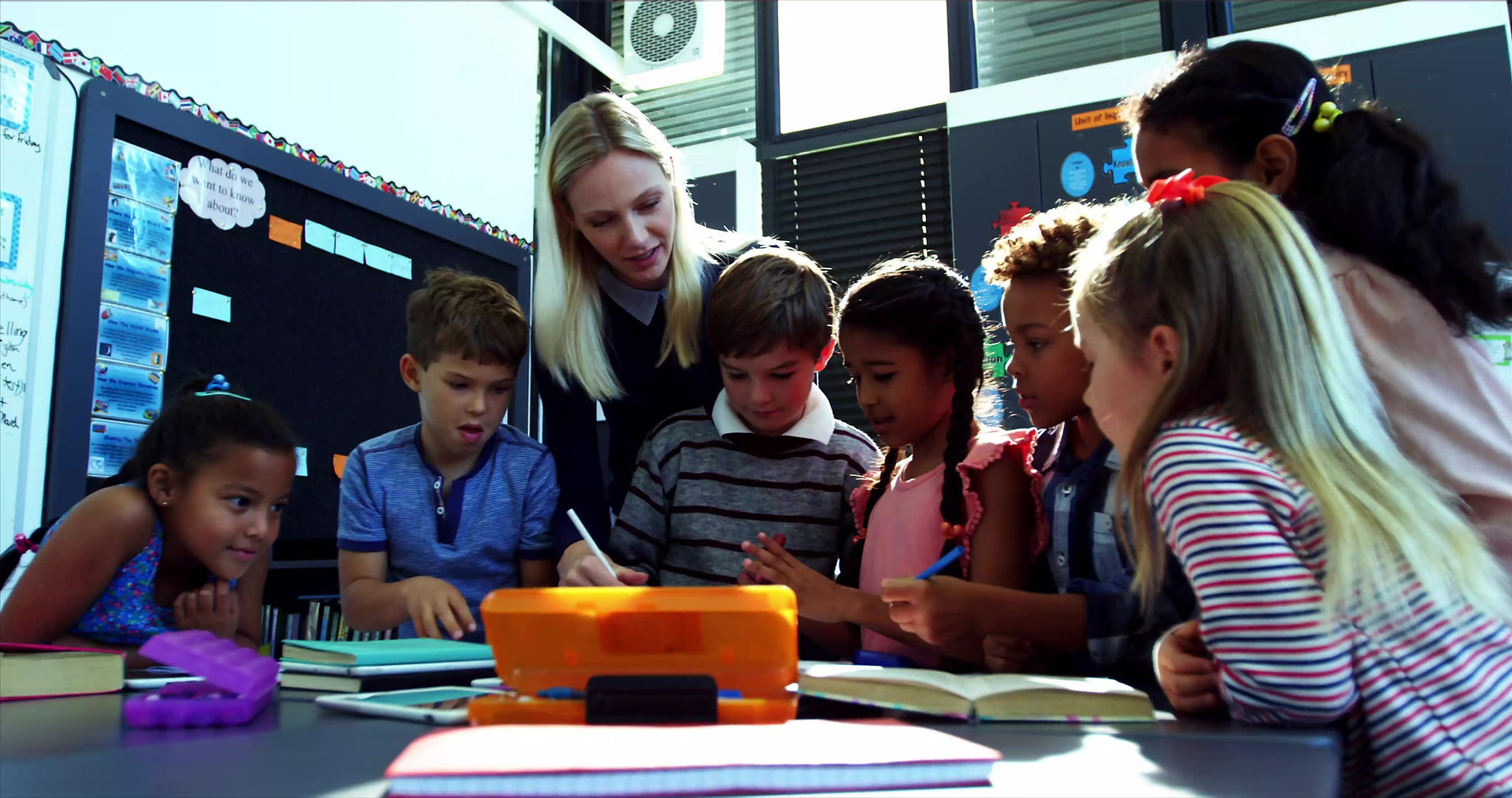
기능을 테스트하려면 라이브 사이트로 이동하세요.
200510
200510
댓글 0개
좋아요
댓글(0)
bottom of page